java图书馆用一个文本文件booklist.txt记录图书的书目,其中包括book1,book2,b
时间: 2023-12-29 17:00:25 浏览: 228
Java图书馆可以使用一个名为booklist.txt的文本文件来记录图书的书目。在这个文件中,可以包含图书的信息,比如书名、作者、出版日期等。例如,可以记录book1、book2和b这几本书的相关信息。
文件booklist.txt 可以使用一种结构化的形式,比如每一行记录一本书的信息,不同信息之间可以使用逗号或者其他分隔符来区分。例如,可以按照以下格式记录书籍信息:
book1, 作者A, 出版日期1, ISBN号1
book2, 作者B, 出版日期2, ISBN号2
b, 作者C, 出版日期3, ISBN号3
当需要查找图书信息时,Java程序可以读取booklist.txt文件,然后根据需要的信息来搜索相应的书目。比如,可以编写一个程序来按照书名搜索,或者按照作者进行筛选。这样,就可以方便地管理和查找图书信息。
另外,Java程序还可以实现对booklist.txt文件的更新功能,比如添加新书籍、删除旧书籍或者修改书籍的信息。这样,图书馆管理人员就可以通过简单的文本文件来管理图书的书目信息,而无需依赖复杂的数据库系统。这种简单而高效的做法,可以帮助图书馆更好地管理图书信息。
相关问题
java代码.试根据已学内容实现一个管理系统(内容不限)。例如图书管理系统,该系统中主要功能包括:(1)查看全部书目;(2)归还书目;(3)添加书目;(4)借阅书目;(5)查找书目。
好的,根据您的需求,我可以为您提供一个简单的图书管理系统的Java代码,实现以下功能:
1. 查看全部书目
2. 归还书目
3. 添加书目
4. 借阅书目
5. 查找书目
代码如下:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class LibraryManagementSystem {
private ArrayList<Book> bookList = new ArrayList<>();
public static void main(String[] args) {
LibraryManagementSystem system = new LibraryManagementSystem();
system.init();
}
public void init() {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("\n欢迎使用图书管理系统!");
System.out.println("1. 查看全部书目");
System.out.println("2. 归还书目");
System.out.println("3. 添加书目");
System.out.println("4. 借阅书目");
System.out.println("5. 查找书目");
System.out.println("0. 退出系统");
System.out.print("请选择操作:");
int choice = scanner.nextInt();
switch (choice) {
case 1:
showAllBooks();
break;
case 2:
returnBook();
break;
case 3:
addBook();
break;
case 4:
borrowBook();
break;
case 5:
searchBook();
break;
case 0:
System.out.println("感谢使用图书管理系统,再见!");
return;
default:
System.out.println("输入有误,请重新选择!");
}
}
}
// 查看全部书目
public void showAllBooks() {
if (bookList.size() == 0) {
System.out.println("暂无书目!");
return;
}
System.out.println("书名\t\t作者\t\t状态");
for (Book book : bookList) {
System.out.println(book.getName() + "\t\t" + book.getAuthor() + "\t\t" + book.getStatus());
}
}
// 归还书目
public void returnBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入书名:");
String name = scanner.nextLine();
for (Book book : bookList) {
if (book.getName().equals(name)) {
if (book.getStatus() == BookStatus.BORROWED) {
book.setStatus(BookStatus.AVAILABLE);
System.out.println("归还成功!");
return;
} else {
System.out.println("该书未被借阅!");
return;
}
}
}
System.out.println("未找到该书!");
}
// 添加书目
public void addBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入书名:");
String name = scanner.nextLine();
System.out.print("请输入作者:");
String author = scanner.nextLine();
Book book = new Book(name, author, BookStatus.AVAILABLE);
bookList.add(book);
System.out.println("添加成功!");
}
// 借阅书目
public void borrowBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入书名:");
String name = scanner.nextLine();
for (Book book : bookList) {
if (book.getName().equals(name)) {
if (book.getStatus() == BookStatus.AVAILABLE) {
book.setStatus(BookStatus.BORROWED);
System.out.println("借阅成功!");
return;
} else {
System.out.println("该书已被借阅!");
return;
}
}
}
System.out.println("未找到该书!");
}
// 查找书目
public void searchBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入书名:");
String name = scanner.nextLine();
for (Book book : bookList) {
if (book.getName().equals(name)) {
System.out.println("书名\t\t作者\t\t状态");
System.out.println(book.getName() + "\t\t" + book.getAuthor() + "\t\t" + book.getStatus());
return;
}
}
System.out.println("未找到该书!");
}
}
class Book {
private String name;
private String author;
private BookStatus status;
public Book(String name, String author, BookStatus status) {
this.name = name;
this.author = author;
this.status = status;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public BookStatus getStatus() {
return status;
}
public void setStatus(BookStatus status) {
this.status = status;
}
}
enum BookStatus {
AVAILABLE,
BORROWED
}
```
该系统中,Book 类表示一本书,包含书名、作者和状态(是否被借阅)。BookStatus 枚举类型表示书的状态。LibraryManagementSystem 类表示图书管理系统,包含了上述要求的各种功能。在 init() 方法中,通过 Scanner 类的输入来选择相应的功能。每个功能的实现细节都在相应的函数中进行了实现。
以上是一个简单的图书管理系统的Java代码,仅供参考。
java创建Person类,属于有name,List<Book>。
在Java中,你可以创建一个名为`Person`的类,它包含两个属性:`name`表示个人姓名,`List<Book>`用于存储一个人拥有的书籍列表。下面是一个简单的例子:
```java
import java.util.ArrayList;
import java.util.List;
public class Person {
private String name; // 姓名
private List<Book> books; // 书籍列表
// 构造函数
public Person(String name) {
this.name = name;
this.books = new ArrayList<>();
}
// getter方法获取name
public String getName() {
return name;
}
// getter方法获取books
public List<Book> getBooks() {
return books;
}
// 添加书籍到books列表的方法
public void addBook(Book book) {
books.add(book);
}
// 其他可能的方法,如删除书籍、打印书籍列表等...
}
// 对于Book类(假设它已经存在),这里省略了
class Book {
// Book类的属性和方法...
}
```
在这个例子中,`Person`类有一个`List<Book>`类型的成员变量,你可以通过构造函数初始化名字,并在后续操作中添加或管理书目。
阅读全文
相关推荐
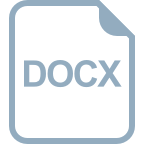
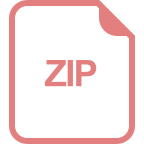
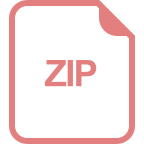













