Java应用程序,其中含有三个类:Triangle(三角形类)、Circle(圆类)和Ladder(梯形类),分别计算它们的面积和周长。
时间: 2024-09-27 20:07:34 浏览: 44
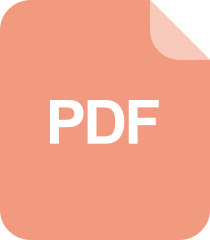
java用接口、多态、继承、类计算三角形和矩形周长及面积的方法

在Java中,创建这样的程序需要每个类都有相应的属性(比如边长、半径等)和计算面积和周长的方法。这里是一个简单的概述:
1. Triangle类:
```java
public class Triangle {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
// 计算面积
public double getArea() {
return 0.5 * base * height;
}
// 计算周长(假设这是一个等腰三角形,只提供一种情况)
public double getPerimeter(double sideLength) {
return 2 * sideLength + base;
}
}
```
2. Circle类:
```java
public class Circle {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
// 计算面积
public double getArea() {
return Math.PI * radius * radius;
}
// 计算周长
public double getPerimeter() {
return 2 * Math.PI * radius;
}
}
```
3. Ladder类(这里假设梯形是对称的,只给出了一种情况):
```java
public class Ladder {
private double topWidth;
private double bottomWidth;
private double height;
public Ladder(double topWidth, double bottomWidth, double height) {
this.topWidth = topWidth;
this.bottomWidth = bottomWidth;
this.height = height;
}
// 计算面积(梯形面积公式:(上底+下底)*高/2)
public double getArea() {
return (topWidth + bottomWidth) * height / 2;
}
// 计算周长(假设梯形对称,只计算一边)
public double getPerimeter() {
return topWidth + bottomWidth;
}
}
```
阅读全文
相关推荐
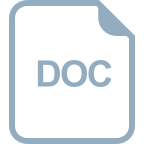
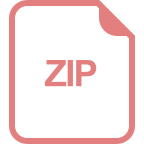
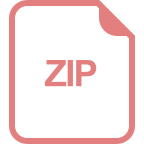
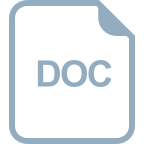
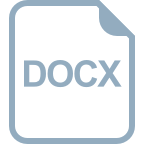
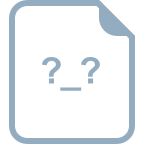
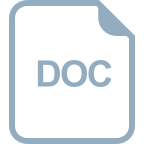
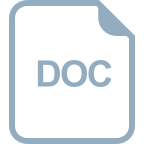
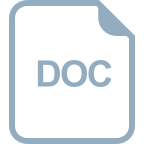

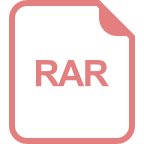
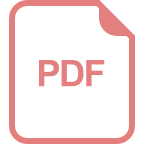
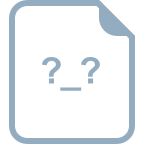
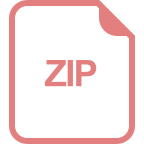
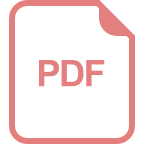
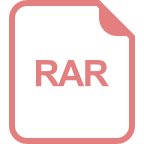
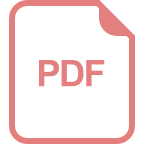