for ind,line in enumerate(wordList): # print('_______________________________________') # print(ind) for i in range(0,50): if 0 == index[ind][0]: fw = open('./cluster' + str(0) + '.txt', 'a+', encoding='utf-8') fw.write(line) # pass else: fw = open('./cluster' + str(1) + '.txt', 'a+', encoding='utf-8') fw.write(line) # pass为什么输出的文件里分词个数都是50的倍数
时间: 2024-04-13 07:28:10 浏览: 557
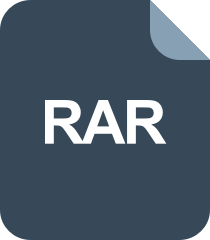
win_is_ind.rar_The Call
输出的文件中分词个数都是50的倍数,是因为在循环中,每次写入文件的时候,是将整个 `line` 写入,而不是根据当前循环的索引 `i` 写入。因此,无论循环多少次,写入的内容都是一样的,都是整个 `line`。如果你想要将每个 `line` 按照50的倍数进行写入,你可以按照以下方式进行修改:
```python
for ind, line in enumerate(wordList):
for i in range(0, 50):
if 0 == index[ind][0]:
fw = open('./cluster' + str(0) + '.txt', 'a+', encoding='utf-8')
fw.write(line[i*50:(i+1)*50])
else:
fw = open('./cluster' + str(1) + '.txt', 'a+', encoding='utf-8')
fw.write(line[i*50:(i+1)*50])
```
这样,每次写入的内容就是 `line` 中从 `i*50` 到 `(i+1)*50` 的部分。这样就可以保证输出的文件中每个分词个数都是50的倍数了。
阅读全文
相关推荐
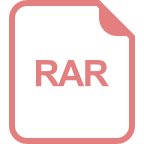
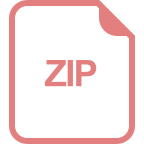
















