wpf中在path路径下建立一个文件夹,文件夹名称存在类型为string的变量A中,然后在666文件夹下建立一个txt文档,里面存入666,txt名称为6
时间: 2024-10-15 21:11:03 浏览: 22
在WPF(Windows Presentation Foundation)环境中创建文件目录并添加文件通常涉及到操作系统级别的操作,而不是直接在XAML或代码-behind中完成。你可以通过C#的System.IO命名空间中的Directory类和File类来进行此类操作。
首先,你需要确保有权限访问路径,并将`string A`作为变量名存储文件夹名称:
```csharp
string path = @"Path\To\Your\Program"; // 指定初始的目录
string folderName = "666"; // 文件夹名称
// 创建文件夹
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path + "\\" + folderName);
}
// 然后在新创建的文件夹下创建txt文件
string fileName = "6.txt";
string filePath = Path.Combine(path + "\\" + folderName, fileName);
using (StreamWriter writer = new StreamWriter(filePath))
{
writer.WriteLine("666");
}
```
上述代码会在指定的程序路径下的"666"文件夹下创建一个名为"6.txt"的文本文件,并写入内容"666"。
相关问题
wpf中,path变量存储一个文件夹地址,点击button在path路径下新建一个文件夹名为A的文件夹
在WPF(Windows Presentation Foundation)中,你可以使用`System.IO.DirectoryInfo`或`System.IO.Path`类来处理文件和目录操作。如果你想创建一个新的文件夹,可以按照以下步骤:
1. 首先,你需要将Path变量转换成`DirectoryInfo`对象,这将让你更容易进行文件夹相关的操作。假设`path`是一个字符串变量存储文件夹地址:
```csharp
string path = @"C:\YourFolderPath";
DirectoryInfo targetDirectory = new DirectoryInfo(path);
```
2. 然后,创建新的文件夹并命名为"A",你可以使用`CreateSubdirectory`方法:
```csharp
string folderName = "A";
DirectoryInfo newFolder = targetDirectory.CreateSubdirectory(folderName);
```
这将在`path`指定的文件夹下创建一个新的名为"A"的子文件夹。
如果你想要在Button的点击事件中完成这个操作,你可以在按钮的Click事件处理器中编写这样的代码:
```csharp
private void Button_Click(object sender, RoutedEventArgs e)
{
string path = // 从Path变量获取文件夹地址...
using (DirectoryInfo targetDirectory = new DirectoryInfo(path))
{
string folderName = "A";
Directory.CreateDirectory(targetDirectory.FullName + "\\" + folderName);
MessageBox.Show($"成功在'{path}\\A'创建了一个新文件夹。");
}
}
```
wpf中从指定路径的txt文档中读取数据,1.txt的640个数据存在2行640列的矩阵变量dataxy的第一行中,2.txt的640个数据存在2行640列的矩阵变量dataxy的第二行中
在WPF中,你可以使用`System.IO.FileStream`和`StreamReader`来读取文本文件中的数据,然后转换为二维矩阵。以下是代码示例,假设你已经有一个名为`dataxy`的二维数组变量:
```csharp
using System.IO;
// 假设你的数据文件路径如下
string path1 = @"path_to_your_directory\1.txt";
string path2 = @"path_to_your_directory\2.txt";
// 初始化矩阵
double[,] dataxy = new double[2, 640];
// 读取1.txt文件并存储到dataxy的第一行
using (FileStream fs1 = new FileStream(path1, FileMode.Open, FileAccess.Read))
{
using (StreamReader sr1 = new StreamReader(fs1))
{
string line;
while ((line = sr1.ReadLine()) != null)
{
String[] values = line.Split(',');
for (int i = 0; i < 640; i++)
{
dataxy[0, i] = Convert.ToDouble(values[i]);
}
}
}
}
// 读取2.txt文件并存储到dataxy的第二行
using (FileStream fs2 = new FileStream(path2, FileMode.Open, FileAccess.Read))
{
using (StreamReader sr2 = new StreamReader(fs2))
{
string line;
while ((line = sr2.ReadLine()) != null)
{
String[] values = line.Split(',');
for (int i = 0; i < 640; i++)
{
dataxy[1, i] = Convert.ToDouble(values[i]);
}
}
}
}
//
阅读全文
相关推荐
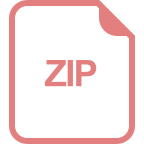
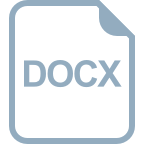
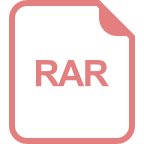
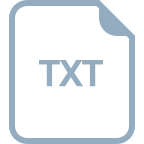
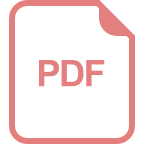
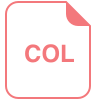
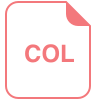
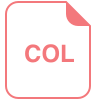
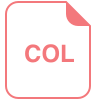
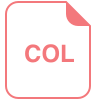






