编写一段C语言代码,要求查询用户输入的MySQL表
时间: 2024-12-23 20:27:18 浏览: 8
在C语言中,我们可以使用MySQL C API来执行SQL查询。下面是一个简单的示例,展示了如何获取用户的输入并查询MySQL表:
```c
#include <stdio.h>
#include <mysql.h>
MYSQL *conn; // MySQL连接指针
// 初始化MySQL连接
void init_mysql_conn(char *host, char *user, char *password, char *database) {
conn = mysql_init(NULL);
if (conn == NULL) {
perror("mysql_init failed");
exit(EXIT_FAILURE);
}
if (mysql_real_connect(conn, host, user, password, database, 0, NULL, 0) == NULL) {
perror("mysql_real_connect failed");
mysql_close(conn);
exit(EXIT_FAILURE);
}
}
// 执行SQL查询
void execute_query(const char *query) {
int result_code = mysql_query(conn, query);
if (result_code != 0) {
fprintf(stderr, "Query failed: %s\n", mysql_error(conn));
return;
}
MYSQL_RES *res = mysql_store_result(conn);
if (res == NULL) {
fprintf(stderr, "No result set for the query.\n");
return;
}
// 遍历查询结果并打印
MYSQL_ROW row;
while ((row = mysql_fetch_row(res))) {
printf("%s\n", row[0]); // 通常这里会根据列的数量调整列索引
}
mysql_free_result(res); // 释放结果集
}
int main() {
char host[] = "localhost";
char user[] = "your_username";
char password[] = "your_password";
char database[] = "your_database";
char tableName[100]; // 假设表名长度不超过100个字符
// 获取用户输入的表名
printf("Enter the name of the table you want to query: ");
fgets(tableName, sizeof(tableName), stdin);
tableName[strlen(tableName) - 1] = '\0'; // 去除换行符
init_mysql_conn(host, user, password, database);
execute_query("SELECT * FROM `" + tableName + "`"); // SQL查询模板
mysql_close(conn);
return 0;
}
```
这个程序首先提示用户输入他们想要查询的表名,然后尝试连接到MySQL服务器并执行查询。请注意,这只是一个基本示例,实际应用中需要处理错误和异常。
阅读全文
相关推荐
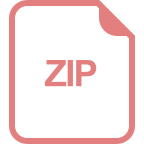
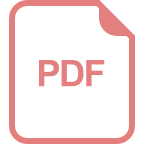
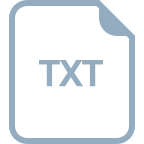









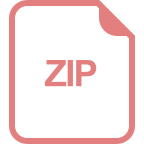
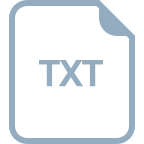
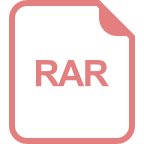
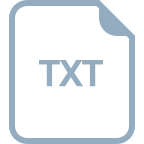
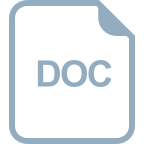
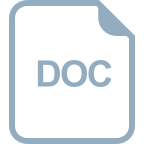
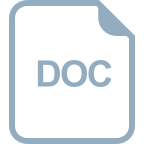