请用c++详细描述把rgb24转换为yuv420
时间: 2024-10-10 21:15:51 浏览: 34
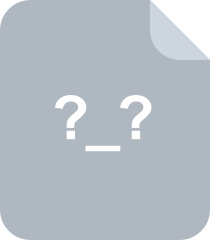
C/C++源码,图像RGB转换为YUV420sp和YUV420sp格式转换为YUV422
RGB24到YUV420是一种常见的颜色空间转换,特别是在视频编码和图像处理中。RGB (Red Green Blue) 是一种加色混合模式,而 YUV (Luma - Chrominance) 则是一种减色混合模式,特别适合于传输和显示。YUV420表示每个像素由一个亮度分量(Y) 和两个 chrominance (Cb and Cr) 分量组成,其中Cb和Cr分量每四个像素共享。
以下是使用C++描述将RGB24(通常是一个字节的红、绿、蓝三个分量)转换为YUV420的一种简单方法:
```cpp
#include <cstdint>
// RGB24 struct representing a pixel in ARGB format (alpha is often ignored)
struct RGB24 {
uint8_t b; // blue
uint8_t g; // green
uint8_t r; // red
};
// YUV420 pixel structure, assuming 8-bit components
struct YUV420 {
uint8_t y; // luma
uint8_t uc[2]; // Cb/Cr packed as two bytes (since they're often downsampled by a factor of 2)
};
// Convert a single RGB24 pixel to YUV420
YUV420 rgbToYuv(RGB24 rgb) {
const int16_t R = static_cast<int16_t>(rgb.r);
const int16_t G = static_cast<int16_t>(rgb.g);
const int16_t B = static_cast<int16_t>(rgb.b);
// Luma calculation
int16_t Y = 17 * R + 17 * G + 24 * B / 32;
// Chrominance calculation (approximate)
int16_t Cr = (R - G) + 128;
int16_t Cb = (B - G) / 2 + 128;
// Pack Cb and Cr into a single byte array for YUV420 format
YUV420 yuv;
yuv.y = static_cast<uint8_t>(Y >> 3); // Shift and truncate to 8 bits
yuv.uc[0] = static_cast<uint8_t>((Cr >> 3) + 16); // Downsample and pack Cb
yuv.uc[1] = static_cast<uint8_t>((Cb >> 2) + 16); // Downsample and pack Cr
return yuv;
}
阅读全文
相关推荐
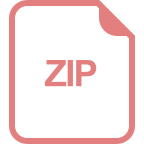
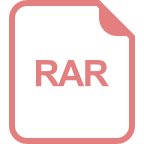
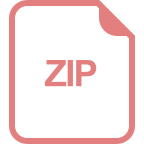
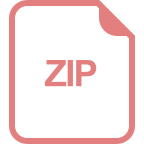

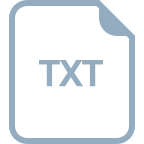
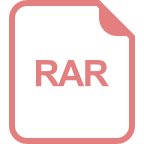
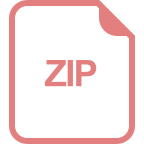
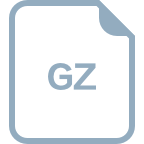
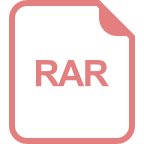
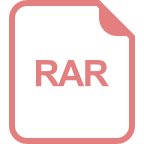
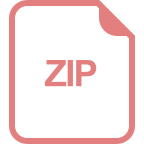
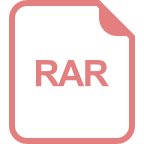
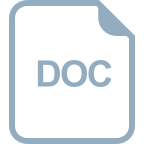
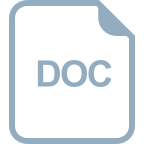
