html给有焦点的表格单元格绑定左右上下键的js代码
时间: 2024-09-17 08:04:48 浏览: 41
在HTML中,你可以通过JavaScript监听键盘事件并结合表格的tabindex属性来实现对有焦点的表格单元格的导航。以下是一个简单的示例:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>表格方向键控制</title>
<style>
table {
border-collapse: collapse;
}
td {
width: 50px;
height: 50px;
border: 1px solid black;
cursor: pointer;
}
</style>
</head>
<body>
<table id="myTable">
<tr>
<td tabindex="0" class="current-cell">1</td>
<td>2</td>
<td>3</td>
</tr>
<tr>
<td>4</td>
<td>5</td>
<td>6</td>
</tr>
<!-- 添加更多行... -->
</table>
<script>
const table = document.getElementById('myTable');
const cells = Array.from(table.getElementsByTagName('td'));
function moveFocus(direction) {
const currentCell = document.querySelector('.current-cell');
let nextCell;
switch (direction) {
case 'up':
if (currentCell.previousElementSibling) {
nextCell = currentCell.previousElementSibling;
} else {
// 如果已在第一行,则移动到最后一列
nextCell = cells[cells.length - 1];
}
break;
case 'down':
if (currentCell.nextElementSibling) {
nextCell = currentCell.nextElementSibling;
} else {
// 如果已在最后一行,则移动到第一列
nextCell = cells[0];
}
break;
case 'left':
if (currentCell.previousSibling && !currentCell.previousElementSibling) { // 非首列
nextCell = currentCell.parentElement.children[currentCell.cellIndex - 1];
} else if (currentCell.previousElementSibling) { // 首列,上移至下一行
nextCell = currentCell.nextElementSibling;
} else {
// 如果在表头,保持不变
return;
}
break;
case 'right':
if (currentCell.nextSibling && !currentCell.nextElementSibling) { // 非尾列
nextCell = currentCell.parentElement.children[currentCell.cellIndex + 1];
} else if (currentCell.nextElementSibling) { // 尾列,下移至上一行
nextCell = currentCell.previousElementSibling;
} else {
// 如果在表尾,保持不变
return;
}
break;
default:
return;
}
currentCell.classList.remove('current-cell');
nextCell.focus();
nextCell.classList.add('current-cell');
}
table.addEventListener('keydown', (e) => {
const keyCode = e.keyCode;
if (keyCode === 37 || keyCode === 39) { // 左右箭头
moveFocus(e.key);
}
// 其他方向键处理...
});
// 初始化焦点在第一个可聚焦单元格
table.firstElementChild.focus();
table.firstElementChild.classList.add('current-cell');
</script>
</body>
</html>
```
这个例子演示了如何使用JavaScript监听`keydown`事件,当用户按下左右箭头时,根据当前选中的单元格切换焦点。注意,为了简洁起见,这里只实现了左右方向的切换,如果你需要处理上下方向,只需添加相应的判断和逻辑即可。
阅读全文
相关推荐
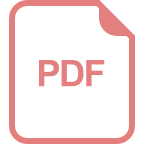
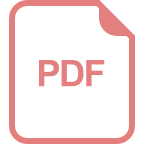
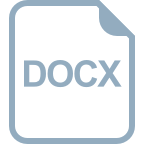




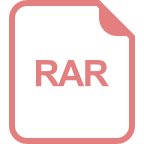
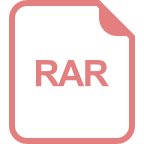
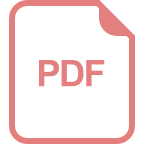
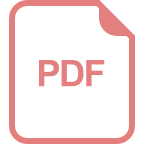
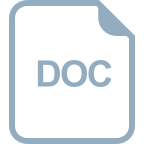
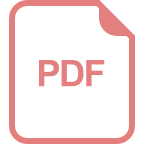
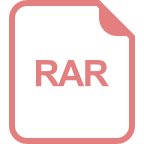
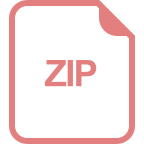
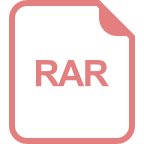
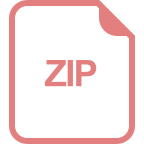


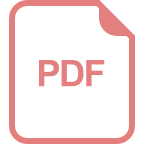