protobuf 读取与存储数据
时间: 2023-09-19 16:11:15 浏览: 233
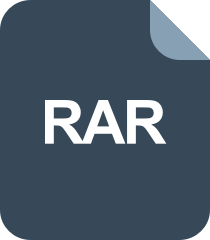
protobuf3.20.1 for android
Protobuf(Protocol Buffers)是一种高效的数据序列化格式,用于在不同的系统之间进行数据交换和存储。下面是关于如何使用Protobuf进行数据的读取和存储的示例:
1. 定义消息格式
在使用Protobuf之前,首先需要定义消息格式。消息格式是使用Protobuf语言定义的,一般以`.proto`为后缀名。
例如,假设我们要存储一个学生的信息,可以创建一个`student.proto`文件,并定义如下消息格式:
```protobuf
syntax = "proto3";
message Student {
string name = 1;
int32 age = 2;
repeated string courses = 3;
}
```
2. 生成代码
定义完消息格式后,需要使用Protobuf编译器生成对应的代码。可以使用官方提供的编译器或者相关库来生成代码。
例如,使用官方提供的编译器来生成Python代码:
```bash
protoc --python_out=. student.proto
```
这将生成一个`student_pb2.py`文件,包含了与消息格式相关的Python类和方法。
3. 序列化数据
在存储数据之前,需要将数据序列化为二进制格式。可以通过创建消息对象并设置字段值来实现。
```python
import student_pb2
student = student_pb2.Student()
student.name = "Alice"
student.age = 20
student.courses.extend(["Math", "English"])
# 序列化为二进制
binary_data = student.SerializeToString()
# 将二进制数据保存至文件
with open("student.bin", "wb") as f:
f.write(binary_data)
```
4. 反序列化数据
在读取数据时,需要将二进制数据反序列化为消息对象。
```python
import student_pb2
# 从文件读取二进制数据
with open("student.bin", "rb") as f:
binary_data = f.read()
# 反序列化为消息对象
student = student_pb2.Student()
student.ParseFromString(binary_data)
# 访问字段值
print(student.name)
print(student.age)
print(student.courses)
```
这样就可以实现Protobuf的数据读取和存储。注意,在使用Protobuf时,双方需要使用相同的消息格式定义和生成的代码。
阅读全文
相关推荐
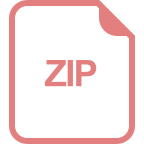
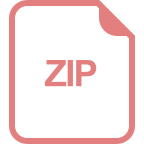

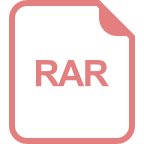
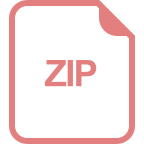
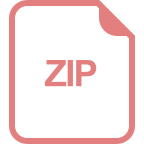
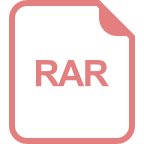
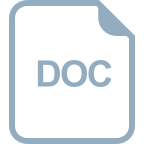
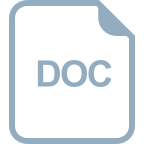
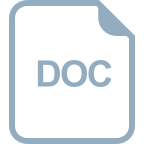
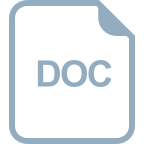
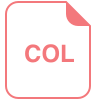
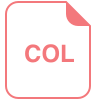




