帮我写一个C++和linux兼容的查找指定文件的函数
时间: 2024-04-14 12:29:11 浏览: 36
当然,下面是一个示例的 C++ 函数,可以在 Linux 系统上查找指定文件:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <dirent.h>
#include <sys/stat.h>
std::vector<std::string> findFiles(const std::string& searchDir, const std::string& fileName) {
std::vector<std::string> result;
DIR* dir;
struct dirent* entry;
struct stat fileInfo;
dir = opendir(searchDir.c_str());
if (dir == nullptr) {
std::cerr << "Error opening directory: " << searchDir << std::endl;
return result;
}
while ((entry = readdir(dir)) != nullptr) {
std::string path = searchDir + "/" + entry->d_name;
if (stat(path.c_str(), &fileInfo) == -1) {
std::cerr << "Error getting file info: " << path << std::endl;
continue;
}
if (S_ISDIR(fileInfo.st_mode)) {
// 忽略目录 . 和 ..
if (std::string(entry->d_name) == "." || std::string(entry->d_name) == "..")
continue;
// 递归搜索子目录
std::vector<std::string> subResult = findFiles(path, fileName);
result.insert(result.end(), subResult.begin(), subResult.end());
} else {
// 检查文件名是否匹配
if (std::string(entry->d_name) == fileName)
result.push_back(path);
}
}
closedir(dir);
return result;
}
int main() {
std::string searchDir = "/path/to/search_dir";
std::string fileName = "filename.txt";
std::vector<std::string> files = findFiles(searchDir, fileName);
if (files.empty()) {
std::cout << "No files found." << std::endl;
} else {
std::cout << "Found files:" << std::endl;
for (const auto& file : files) {
std::cout << file << std::endl;
}
}
return 0;
}
```
将上述代码保存为一个名为 `find_files.cpp` 的文件,并使用以下命令进行编译:
```
g++ find_files.cpp -o find_files
```
然后运行生成的可执行文件 `find_files` 即可在指定目录下查找指定文件。请确保将 `"/path/to/search_dir"` 替换为实际的搜索目录路径,并将 `"filename.txt"` 替换为实际的文件名。
这个函数使用了 `<iostream>`, `<string>`, `<vector>`, `<dirent.h>` 和 `<sys/stat.h>` 头文件,以及相关的 C++ 标准库和系统调用函数来实现文件搜索功能。它会递归搜索指定目录及其子目录中的文件,并将匹配的文件路径存储在一个 `std::vector` 中返回。
相关推荐
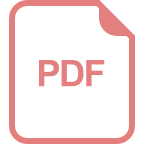
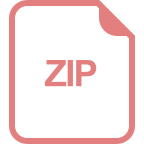
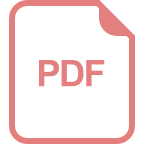














