用C语言编程用户登陆程序 (1)输入用户名和密码 (2)判断用户名和密码是否正确( ‘name==dd’,'passwd=‘nn’ ) (3)登陆次数仅有三次,如果超过三次机会,报错
时间: 2024-09-07 21:03:31 浏览: 135
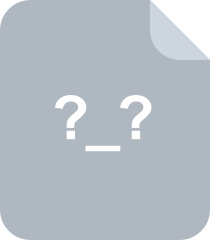
输入用户名密码的程序c++
在C语言中编写一个简单的用户登录程序可以分为以下几个步骤:
1. 定义变量和结构体(如果需要的话):
```c
#include <stdio.h>
#include <string.h>
// 用户信息结构体
typedef struct {
char username[50];
char password[50];
} User;
// 登录尝试计数器
int login_attempts = 0;
```
2. 主函数`main()`实现:
```c
int main() {
const char *username_pattern = "dd";
const char *password_pattern = "nn";
while (login_attempts < 3) { // 设置最多三次尝试
printf("请输入用户名:");
fgets(User.username, sizeof(User.username), stdin);
scanf("%*[^\n]"); // 清除多余的换行符
printf("请输入密码:");
fgets(User.password, sizeof(User.password), stdin);
scanf("%*[^\n]");
// 检查用户名和密码是否匹配
if (strcmp(User.username, username_pattern) == 0 && strcmp(User.password, password_pattern) == 0) {
printf("登录成功!\n");
break; // 如果匹配,退出循环
} else {
printf("用户名或密码错误,已尝试 %d 次。\n", login_attempts + 1);
login_attempts++;
}
}
// 超过三次尝试的情况
if (login_attempts == 3) {
printf("抱歉,您已经达到了最大尝试次数,无法继续登录。\n");
}
return 0;
}
```
在这个程序中,我们使用了`fgets()`读取用户的输入,并通过`strcmp()`函数比较输入的用户名和密码与预设模式。每次尝试失败时,我们会增加登录尝试次数直到达到上限。
阅读全文
相关推荐
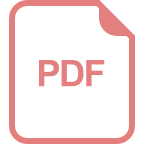
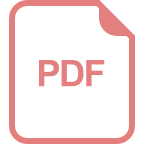















