写一段java代码输出一组公司员工的信息列表,其中员工具备以下特征:雇员:员工编号、姓名、工资;经理:员工编号、姓名、工资、管理部门;总监:员工编号、姓名、工资、津贴;
时间: 2024-11-24 19:42:49 浏览: 7
以下是一个简单的Java代码片段,它使用类和数组来存储公司的员工信息,并根据职位类型(雇员、经理、总监)展示不同的属性:
```java
import java.util.ArrayList;
class Employee {
private int id;
private String name;
private double salary;
public Employee(int id, String name, double salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
public int getId() { return id; }
public String getName() { return name; }
public double getSalary() { return salary; }
// 对雇员而言,无需显示管理部门或津贴
@Override
public String toString() {
return "员工编号:" + id + ",姓名:" + name + ",薪资:" + salary;
}
}
class Manager extends Employee {
private String department;
public Manager(int id, String name, double salary, String department) {
super(id, name, salary);
this.department = department;
}
public String getDepartment() { return department; }
@Override
public String toString() {
return super.toString() + ",部门:" + department;
}
}
class Director extends Employee {
private double bonus;
public Director(int id, String name, double salary, double bonus) {
super(id, name, salary);
this.bonus = bonus;
}
public double getBonus() { return bonus; }
@Override
public String toString() {
return super.toString() + ",津贴:" + bonus;
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Employee> employees = new ArrayList<>();
// 添加雇员
employees.add(new Employee(1, "张三", 5000));
employees.add(new Employee(2, "李四", 6000));
// 添加经理
employees.add(new Manager(3, "王五", 8000, "市场部"));
employees.add(new Manager(4, "赵六", 9000, "技术部"));
// 添加总监
employees.add(new Director(5, "孙七", 12000, 3000));
for (Employee e : employees) {
System.out.println(e);
}
}
}
```
这段代码首先定义了三个类:`Employee`(基础员工)、`Manager`(经理)和`Director`(总监),然后在`Main`类的`main`方法中创建了一组员工对象,并将它们添加到`ArrayList`中。最后遍历数组并打印每个员工的信息。
阅读全文
相关推荐
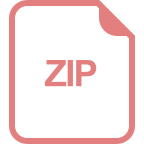
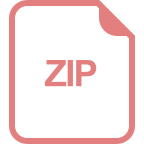
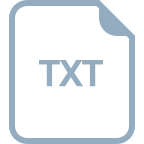















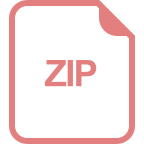
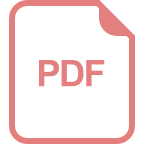