c++如何创建单链表
时间: 2024-10-17 18:05:13 浏览: 21
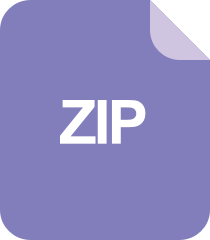
使用C++实现单链表的基本操作:1、创建单链表2、遍历单链表3、单链表插入4、删除单链表5、判断是否为空6、单链表的

在C++中,创建单链表通常涉及以下几个步骤:
1. 定义节点结构:首先,你需要定义一个`Node`结构体或类,它包含数据域`data`以及指向下一个节点的指针`next`。
```cpp
struct Node {
int data; // 数据域
Node* next; // 指向下一个节点的指针
};
```
2. 创建链表头结点:如果你还没有链表,通常会先创建一个空的头结点,表示链表开始。
```cpp
Node* head = nullptr;
```
3. 插入节点:你可以编写一个函数来插入新节点到链表的任意位置,例如在头部、尾部或中间。
```cpp
void insertAtFront(Node** head, int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 插入在尾部
void append(Node** head, int value) {
if (*head == nullptr) {
*head = new Node{value, nullptr};
return;
}
Node* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node{value, nullptr};
}
```
4. 遍历链表:通过改变当前节点并访问`next`属性来遍历整个链表。
```cpp
void printList(Node* head) {
Node* currentNode = head;
while (currentNode != nullptr) {
std::cout << currentNode->data << " ";
currentNode = currentNode->next;
}
std::cout << "\n";
}
```
阅读全文
相关推荐
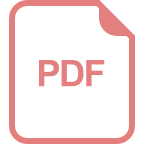
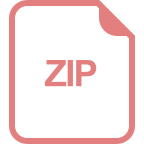


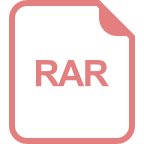
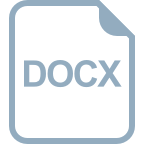
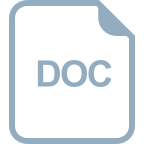
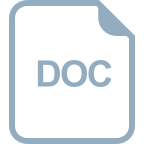
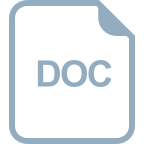
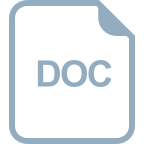
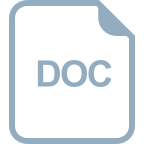
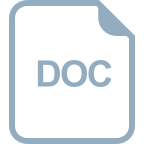
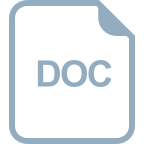



