cstring转unsigned short int
时间: 2023-08-25 17:05:31 浏览: 210
要将一个C字符串转换为无符号短整数(unsigned short int),你可以使用标准库函数`strtoul`或者`sscanf`。下面是使用这两种方法的示例代码:
使用`strtoul`函数:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char str[] = "12345";
unsigned short int num = (unsigned short int)strtoul(str, NULL, 0);
printf("%hu\n", num);
return 0;
}
```
使用`sscanf`函数:
```c
#include <stdio.h>
int main() {
char str[] = "12345";
unsigned short int num;
sscanf(str, "%hu", &num);
printf("%hu\n", num);
return 0;
}
```
这两种方法都将字符串转换为无符号长整数,然后将其强制转换为无符号短整数。在这里,输入字符串"12345"将被转换为等效的无符号短整数12345,并打印出来。
相关问题
试采用C++编写一小程序,要求:定义函数 void uitos1(unsigned short int i, char* var), 函数功能:实现将无符号短整形变量中的数据转换为字符串形式并保存至实参指定的字符数组里。
下面是一个简单的C++程序示例,它定义了一个名为uitos1的函数,该函数实现了从无符号短整型(i)到字符串(var)的转换:
```cpp
#include <cstring> // 引入cstring库用于操作字符串
// 定义函数uitos1
void uitos1(unsigned short int i, char* var) {
// 创建一个临时字符串,用于存储无符号短整型转换后的值
char temp[6]; // 短整型最多6位二进制数,加上前导0和空终止符共7个字符
// 将数字转换为字符串
std::snprintf(temp, sizeof(temp), "%hu", i);
// 移除字符串末尾的空终止符(如果存在)
if (temp[strlen(temp)-1] == '\0') {
temp[strlen(temp)-1] = '\0'; // 如果最后一个字符是'\0'
}
// 将转换后的字符串复制到用户指定的字符数组var中
strcpy(var, temp);
}
int main() {
unsigned short int num = 1234;
char str[10]; // 假设用户提供的字符数组大小足够
// 调用uitos1函数
uitos1(num, str);
// 输出转换后的字符串
std::cout << "数值 " << num << " 转换为字符串为: " << str << std::endl;
return 0;
}
```
在这个例子中,uitos1函数首先创建一个临时字符串,然后使用`std::snprintf`函数格式化输入的整数为无符号十六进制表示,并自动添加适当的前导零。接着,如果需要,去掉多余的空终止符,最后将结果复制到用户提供的字符数组`var`中。
MFC中怎么把unsigned short*保存为raw
在 MFC 中,你可以使用 CFile 类来保存 unsigned short 数组为 raw 文件。下面是一个简单的示例代码,展示了如何实现这个功能:
```cpp
#include <afx.h>
bool SaveUnsignedShortToRaw(const unsigned short* data, int width, int height, const CString& filePath)
{
CFile file;
if (file.Open(filePath, CFile::modeCreate | CFile::modeWrite))
{
// 计算数据字节数
int dataSize = width * height * sizeof(unsigned short);
// 写入数据到文件
file.Write(data, dataSize);
// 关闭文件
file.Close();
return true;
}
return false;
}
```
你可以调用 `SaveUnsignedShortToRaw` 函数来保存 unsigned short 数组为 raw 文件。需要传入数据指针、图像的宽度和高度,以及保存的文件路径。该函数打开一个文件并以二进制写入模式打开,然后将数据写入文件,最后关闭文件。
请注意,上述示例中使用了 `CString` 类来表示文件路径。你可以根据实际情况调整参数类型。
希望对你有所帮助!如有更多问题,请随时提问。
相关推荐
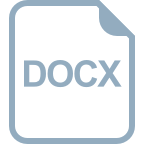
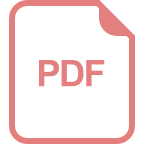












