如果文档中含有表格,该如何解析并提取,请给出Python代码示例
时间: 2024-10-06 14:05:03 浏览: 27
如果你的文档中确实包含了表格,并且你想通过Python来提取这些表格的内容,无论是Word还是PDF,都需要借助不同的库。这里以常见的工具举例:
**处理Word文档中的表格**(`python-docx`库):
```python
from docx import Document
from docx.table import Table
def parse_word_table(file_path):
doc = Document(file_path)
tables = doc.tables
parsed_tables = []
for table in tables:
rows = []
for row in table.rows:
cells = [cell.text.strip() for cell in row.cells]
rows.append(cells)
parsed_tables.append(rows)
return parsed_tables
word_file_path = 'your_word_file_with_tables.docx'
parsed_word_tables = parse_word_table(word_file_path)
```
**处理PDF中的表格**(`tabula-py`库,假设表格位于PDF第一页):
```python
import tabula
def parse_pdf_table(file_path):
table = tabula.read_pdf(file_path, pages='1', output_format='dataframe') # 读取第一张表
return table
pdf_file_path = 'your_pdf_file_with_tables.pdf'
parsed_pdf_table = parse_pdf_table(pdf_file_path)
```
请注意,`tabula-py`默认会尝试识别PDF中的表格并转换为Pandas DataFrame,这在大多数情况都能很好地工作。如果表格复杂或者位置不固定,可能需要调整参数或使用其他库如`camelot`。
**合并并处理结果**:
```python
if parsed_word_tables and parsed_pdf_table:
all_tables = parsed_word_tables + parsed_pdf_table
# 对表格数据进行清洗(例如去除空值、统一列名等)
cleaned_tables = [{k: v for k, v in row.items() if v} for row in all_tables]
# 最后,cleaned_tables 就是你想要的表格数据列表
```
阅读全文
相关推荐
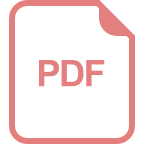
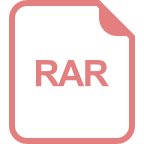
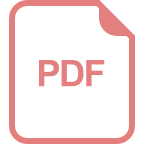
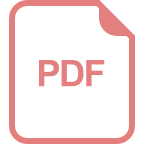














