平面坐标系中随机产生十个点,求哪两点之间距离最短java
时间: 2024-10-09 11:13:13 浏览: 18
在Java中,为了在平面坐标系中随机生成十个点并找出两点间最短距离,你可以按照以下步骤操作:
1. 首先,创建一个Point类,包含x和y坐标属性以及构造函数、toString方法等。
```java
import java.util.Random;
class Point {
double x;
double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
@Override
public String toString() {
return "Point{" +
"x=" + x +
", y=" + y +
'}';
}
}
```
2. 使用Random类生成随机的x和y坐标。
```java
public static List<Point> generatePoints(int count) {
Random random = new Random();
List<Point> points = new ArrayList<>();
for (int i = 0; i < count; i++) {
points.add(new Point(random.nextDouble(), random.nextDouble()));
}
return points;
}
```
3. 创建一个方法计算任意两个点之间的距离,并找到最短距离。这里可以使用`Math.hypot()`来简化计算过程。
```java
private static double distance(Point a, Point b) {
return Math.hypot(a.x - b.x, a.y - b.y);
}
public static Point findClosestPair(List<Point> points) {
if (points.size() <= 1) {
throw new IllegalArgumentException("At least two points are required.");
}
// 初始化最远和最近的距离,假设第一个点与第二个点间的距离是最小值
double shortestDistance = distance(points.get(0), points.get(1));
Point closestPair = new Point(points.get(0), points.get(1));
// 使用双指针法遍历列表
for (int i = 0; i < points.size() - 1; i++) {
for (int j = i + 1; j < points.size(); j++) {
double currentDistance = distance(points.get(i), points.get(j));
if (currentDistance < shortestDistance) {
shortestDistance = currentDistance;
closestPair = new Point(points.get(i), points.get(j));
}
}
}
return closestPair;
}
```
4. 最后,测试这个方法。
```java
public static void main(String[] args) {
int pointCount = 10;
List<Point> points = generatePoints(pointCount);
Point closestPair = findClosestPair(points);
System.out.println("Closest pair: " + closestPair);
System.out.println("Shortest distance: " + shortestDistance);
}
```
阅读全文
相关推荐
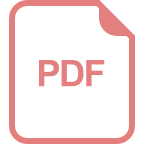


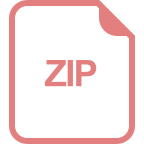
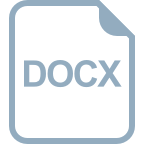
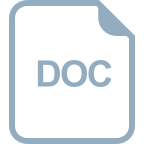
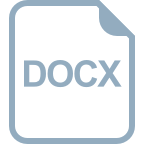
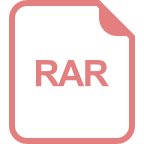
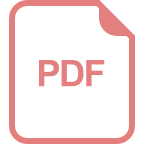
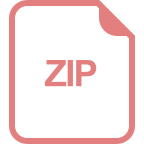
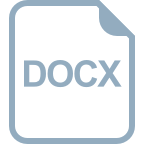
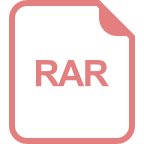

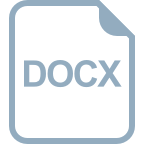
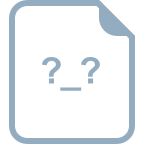
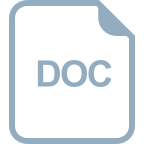