Python绘制彩球飘飘
时间: 2024-05-27 14:06:51 浏览: 170
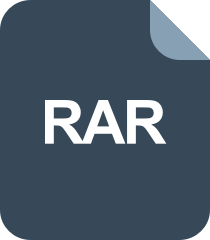
制作彩旗飘扬的效果的一个工具
可以使用Python的turtle库来实现绘制彩球飘飘的效果。以下是一个简单的实现方式:
```
import turtle
import random
# 设置画笔颜色列表
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
# 设置窗口大小和背景色
turtle.setup(width=600, height=600)
turtle.bgcolor("black")
# 创建小球类
class Ball(turtle.Turtle):
def __init__(self, color):
super().__init__(shape="circle")
self.color(color)
self.penup()
self.speed(0)
self.goto(random.randint(-250, 250), random.randint(-250, 250))
self.dx = random.randint(-3, 3)
self.dy = random.randint(-3, 3)
def move(self):
x = self.xcor() + self.dx
y = self.ycor() + self.dy
# 碰到边界后反弹
if x < -290 or x > 290:
self.dx *= -1
if y < -290 or y > 290:
self.dy *= -1
self.goto(x, y)
# 创建小球对象列表
balls = [Ball(color) for color in colors]
# 循环移动小球对象并绘制
while True:
for ball in balls:
ball.move()
turtle.update()
```
上述代码会创建6个彩色小球并在窗口内移动。每个小球都有随机的初始位置和速度,并且碰到边界后会反弹。程序会一直循环运行,直到手动关闭窗口。
阅读全文
相关推荐
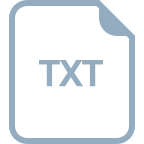
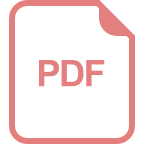
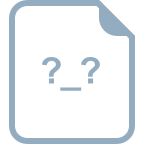
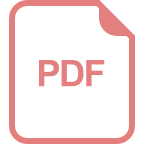
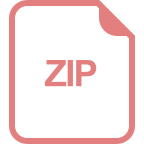
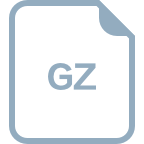
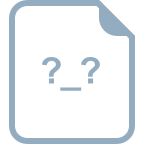
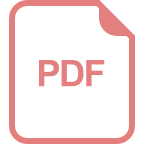
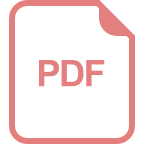
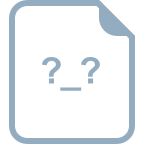
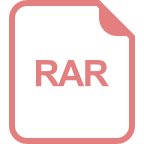