python使用ffmpeg推流
时间: 2023-08-27 11:08:58 浏览: 66
要使用Python进行FFmpeg推流,你可以使用`subprocess`模块来调用FFmpeg命令。下面是一个简单的示例代码:
```python
import subprocess
def start_streaming(input_file, output_url):
command = ['ffmpeg', '-i', input_file, '-c:v', 'libx264', '-preset', 'ultrafast', '-f', 'flv', output_url]
process = subprocess.Popen(command)
process.wait()
input_file = 'input.mp4'
output_url = 'rtmp://example.com/live/stream_key'
start_streaming(input_file, output_url)
```
在这个示例中,`input_file`是要推流的视频文件路径,`output_url`是要推流到的URL地址。你可以根据实际情况修改这两个参数。
注意,你需要在你的系统上安装FFmpeg,并将其添加到系统环境变量中,以便Python能够找到它。
相关问题
python结合ffmpeg推流
要在Python中结合FFmpeg进行推流,你可以使用subprocess模块来调用FFmpeg命令。以下是一个简单的示例:
```python
import subprocess
def start_streaming(input_file, output_url):
ffmpeg_cmd = [
'ffmpeg',
'-i', input_file,
'-c:v', 'copy',
'-c:a', 'aac',
'-f', 'flv',
output_url
]
subprocess.Popen(ffmpeg_cmd)
# 示例调用
input_file = 'input.mp4'
output_url = 'rtmp://example.com/live/stream_key'
start_streaming(input_file, output_url)
```
在这个示例中,`input_file`是你要推流的本地文件路径,`output_url`是你要推流到的目标URL。在`ffmpeg_cmd`中,我们使用了一些常见的FFmpeg命令参数,包括`-i`指定输入文件,`-c:v`和`-c:a`指定视频和音频编码器,`-f`指定输出格式为FLV。
通过使用`subprocess.Popen`函数,我们可以在Python中调用FFmpeg命令,并开始推流。
请确保你已经安装了FFmpeg,并且将其添加到系统的环境变量中,以便在Python中可以访问到它。
python ffmpeg 推流
要使用 Python 进行 ffmpeg 推流,你需要安装 ffmpeg 库并在 Python 代码中使用 subprocess 模块来执行 ffmpeg 命令。以下是一个示例代码:
```python
import subprocess
# ffmpeg 推流命令
ffmpeg_command = "ffmpeg -re -i input.mp4 -c:v libx264 -preset veryfast -b:v 3000k -maxrate 3000k -bufsize 6000k -pix_fmt yuv420p -g 50 -c:a aac -b:a 128k -ac 2 -f flv rtmp://your-streaming-server.com/stream-key"
# 执行命令
subprocess.call(ffmpeg_command, shell=True)
```
在上面的代码中,你需要将输入文件 `input.mp4` 和推流地址 `rtmp://your-streaming-server.com/stream-key` 替换为你自己的。`-re` 参数表示输入文件以实时模式读取,`-c:v libx264` 表示使用 H.264 视频编码器,`-preset veryfast` 表示使用快速编码速度,`-b:v 3000k` 表示视频比特率为 3000kbps,`-maxrate 3000k` 表示视频最大比特率为 3000kbps,`-bufsize 6000k` 表示视频缓存大小为 6000kb,`-pix_fmt yuv420p` 表示使用 YUV420P 色彩空间,`-g 50` 表示视频帧组间隔为 50,`-c:a aac` 表示使用 AAC 音频编码器,`-b:a 128k` 表示音频比特率为 128kbps,`-ac 2` 表示音频通道数为 2,`-f flv` 表示输出格式为 FLV。
相关推荐
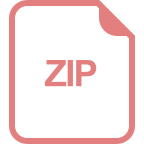
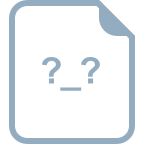












