利用Hadoop处理租房数据分析
时间: 2024-12-27 22:22:11 浏览: 8
### 使用Hadoop处理和分析租房数据
#### 数据收集与预处理
为了有效地使用Hadoop进行租房数据分析,首先需要构建一个可靠的数据管道来获取并清理原始数据。这通常涉及网络爬虫技术的应用,用于从各种在线平台抓取最新的房源列表[^3]。
```python
import requests
from bs4 import BeautifulSoup
def fetch_rental_data(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 假设页面上的每条记录都包含在一个特定类别的div标签内
entries = []
for entry in soup.find_all('div', class_='listing'):
title = entry.find('h2').text.strip()
price = float(entry.select_one('.price').text.replace('$',''))
location = entry.select_one('.location').text.strip()
entries.append({
"title": title,
"price": price,
"location": location
})
return entries
```
#### 存储结构化数据至HDFS
一旦获得了初步的租房信息集合之后,则需将其转换成适合长期存储的形式,并上传到分布式文件系统(HDFS),以便后续阶段能够高效访问这些资料。此过程可能涉及到CSV或JSON格式的选择,具体取决于实际需求[^1]。
```bash
# 将本地文件上传到HDFS指定路径下
$ hadoop fs -put /local/path/to/file.csv /user/hdfs/rental-data/
```
#### 执行MapReduce作业
当所有必要的输入都已经准备就绪时,在集群上启动MapReduce任务来进行大规模计算工作变得至关重要。例如,可以通过编写自定义Mapper和Reducer函数来统计不同地区内的平均房租水平:
```java
// Mapper.java
public static class AvgPriceMapper extends MapReduceBase implements Mapper<LongWritable, Text, Text, DoubleWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, OutputCollector<Text, DoubleWritable> output, Reporter reporter) throws IOException {
String line = value.toString();
String[] parts = line.split(",");
double price = Double.parseDouble(parts[1]);
String area = parts[2];
word.set(area);
output.collect(word, new DoubleWritable(price));
}
}
// Reducer.java
public static class AvgPriceReducer extends MapReduceBase implements Reducer<Text, DoubleWritable, Text, DoubleWritable> {
public void reduce(Text key, Iterator<DoubleWritable> values, OutputCollector<Text, DoubleWritable> output, Reporter reporter) throws IOException {
int count = 0;
double sum = 0;
while(values.hasNext()){
sum += ((DoubleWritable)values.next()).get();
++count;
}
if(count != 0){
output.collect(key, new DoubleWritable(sum/count));
}else{
System.out.println("No data found");
}
}
}
```
#### 结果展示与可视化
最后一步是将得到的结果呈现给最终用户。考虑到用户体验的重要性,建议开发一套基于Web界面的应用程序,允许查询、筛选以及图形化的表示形式,从而使得复杂的信息更加直观易懂。Django框架加上前端图表库(如ECharts)将是不错的选择之一。
阅读全文
相关推荐
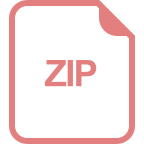
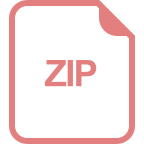
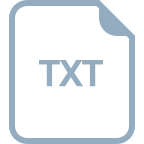
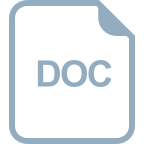
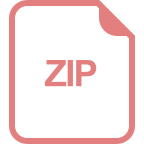
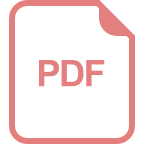
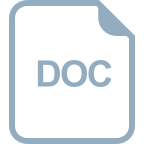
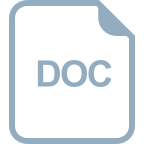
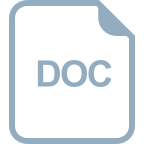


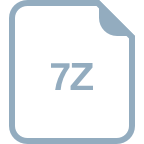
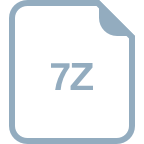
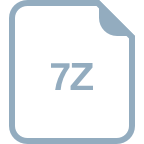
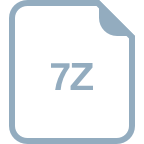
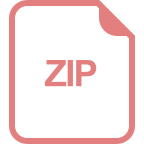
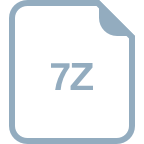