使用android HID 相关代码 使用上面的描述符 发给电脑
时间: 2024-04-16 10:24:05 浏览: 11
以下是一个简单的示例代码,用于在安卓设备上使用Android HID API发送报告描述符给电脑:
```java
import android.hardware.usb.UsbDevice;
import android.hardware.usb.UsbDeviceConnection;
import android.hardware.usb.UsbEndpoint;
import android.hardware.usb.UsbInterface;
import android.hardware.usb.UsbManager;
import java.util.HashMap;
public class MainActivity extends AppCompatActivity {
private static final int VENDOR_ID = 0x1234; // 你的设备供应商ID
private static final int PRODUCT_ID = 0x5678; // 你的设备产品ID
private UsbManager usbManager;
private UsbDevice usbDevice;
private UsbInterface usbInterface;
private UsbDeviceConnection usbDeviceConnection;
private UsbEndpoint outEndpoint;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
usbManager = (UsbManager) getSystemService(Context.USB_SERVICE);
findUsbDevice();
openUsbDevice();
sendReportDescriptor();
}
private void findUsbDevice() {
HashMap<String, UsbDevice> deviceList = usbManager.getDeviceList();
for (UsbDevice device : deviceList.values()) {
if (device.getVendorId() == VENDOR_ID && device.getProductId() == PRODUCT_ID) {
usbDevice = device;
break;
}
}
}
private void openUsbDevice() {
usbInterface = usbDevice.getInterface(0);
usbDeviceConnection = usbManager.openDevice(usbDevice);
usbDeviceConnection.claimInterface(usbInterface, true);
outEndpoint = usbInterface.getEndpoint(0);
}
private void sendReportDescriptor() {
byte[] reportDescriptor = {
// 在这里插入你修改后的报告描述符
};
int transferResult = usbDeviceConnection.bulkTransfer(outEndpoint, reportDescriptor, reportDescriptor.length, 0);
if (transferResult < 0) {
// 发送失败的处理逻辑
} else {
// 发送成功的处理逻辑
}
}
}
```
请确保在 AndroidManifest.xml 文件中添加了 USB 权限:
```xml
<uses-permission android:name="android.permission.USB_PERMISSION" />
```
注意,以上代码仅提供了发送报告描述符的基本框架,具体的实现可能因设备和操作系统版本而异。你需要根据你的设备和需求进行适当的调整和扩展。如果你有任何问题,请随时提问。
相关推荐
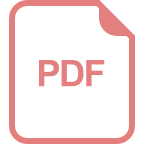














