如何在C++中定义并使用一个仿射函数(也称为操作符重载函数)?
时间: 2024-12-12 21:21:30 浏览: 9
在C++中,可以使用运算符重载(operator overloading)来定义一个仿射函数,也就是自定义某个运算符的行为。对于线性代数中的仿射变换,我们通常会重载`+`和`*`运算符。这里是一个简单的例子,假设我们有一个仿射变换矩阵类`AffineTransformation`:
```cpp
class AffineTransformation {
private:
std::vector<std::vector<double>> matrix; // 代表变换矩阵
public:
// 初始化一个单位矩阵
AffineTransformation() : matrix(3, std::vector<double>(3, 1)) {}
// 运算符重载:加法
AffineTransformation operator+(const AffineTransformation& other) const {
AffineTransformation result = *this;
for (size_t i = 0; i < 3; ++i) {
for (size_t j = 0; j < 3; ++j) {
result.matrix[i][j] += other.matrix[i][j];
}
}
return result;
}
// 运算符重载:点乘(用于缩放)
AffineTransformation operator*(double scalar) const {
AffineTransformation result;
result.matrix = matrix;
for (auto& row : result.matrix)
for (auto& elem : row)
elem *= scalar;
return result;
}
// 可视化方法,方便打印
void print() const {
for (const auto& row : matrix)
for (const auto& elem : row)
std::cout << elem << " ";
std::cout << '\n';
}
};
```
然后你可以这样使用这个仿射变换:
```cpp
int main() {
AffineTransformation t1(AffineTransformation::Identity());
t1.print(); // 输出单位矩阵
t1 = t1 + AffineTransformation({1, 0, 0}, {0, 2, 0}, {0, 0, 3}); // 应该相当于左乘变换矩阵
t1.print();
t1 = 2 * t1; // 应该相当于右乘以2
t1.print();
return 0;
}
```
阅读全文
相关推荐





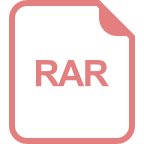








