在C#编程中,如何将格里高利历日期转换为儒略历日期?
时间: 2024-10-26 10:04:00 浏览: 51
在C#中,如果你需要将格里高利历(即公历)日期转换为儒略历日期,可以利用.NET Framework提供的DateTime类和历史纪元日期之间的转换。然而,由于从公元1582年10月4日到10月15日之间有一个约7天的时间跳跃(即著名的“教皇朱斯提尼安二世闰”,因为当时引入了格里高利历),直接的转换可能会有些复杂。
一种常见的做法是先获取当前日期的Unix时间戳(自1970年1月1日以来的秒数),然后计算出对应的儒略历日期。你可以使用以下步骤:
1. 获取公历日期的Unix时间戳(UnixTimestamp):
```csharp
long unixTimestamp = DateTime.UtcNow.ToUniversalTime().Ticks / TimeSpan.TicksPerSecond;
```
2. 使用儒略历的日历年份、月份和日期公式计算儒略日(Julian Day Number):
- 通常使用的公式包括对世纪年份的调整,以及考虑到1582年之前的差异。这个过程可能涉及到复杂的数学运算。
3. 将儒略日转换为儒略历日期。不过,.NET框架本身并没有提供直接将Unix时间戳转换为儒略历的功能,因此你可能需要自行编写函数来进行这个计算。
需要注意的是,儒略历并不是简单地每年加一,特别是涉及到公元前的年份和过渡时期,所以处理起来相对复杂。如果你只是在软件开发中做一般性的日期转换,那么直接使用公历通常是更便捷的做法。
相关问题
你知道你生日那天是星期几吗,你知道你爸爸生日那天是星期几吗?你也许会说:可以查万年历啊。然而,不查万年历你能计算出来吗? C语言编程序实现:输入任意一个日期的年、月、日的值,求出从公元1年1月1日到该日期前一年的年末总共有多少天,到该日期前一个月的月末总共有多少天,到这一天总共有多少天,并求出这一天是星期几(用英文表示)。 假定从公元第一天开始,就实施格里高利历法,并且公元1年1月1日为星期一。格里高利历法的置闰规则是400年97闰,也可以概括为:四闰百不闰,四百闰。 提示:可以将每个月的天数存入一个数组中。 输入格式: 输入年、月、日的值,数据之间以空格分隔。
好的,我会尽力回答你的问题。这个问题涉及到日期、闰年等知识,需要用到一些计算方法。以下是 C 语言编写的程序实现:
```c
#include <stdio.h>
// 判断是否为闰年
int isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return 1;
} else {
return 0;
}
}
// 计算该年前一年年末总共有多少天
int daysBeforeYear(int year, int month, int day) {
int days = 0;
int leap = isLeapYear(year - 1); // 前一年是否为闰年
int monthDays[] = {31, 28 + leap, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每个月的天数
for (int i = 0; i < month - 1; i++) {
days += monthDays[i];
}
days += day - 1;
return days;
}
// 计算该月前一个月月末总共有多少天
int daysBeforeMonth(int year, int month, int day) {
int days = 0;
int leap = isLeapYear(year); // 是否为闰年
int monthDays[] = {31, 28 + leap, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每个月的天数
for (int i = 0; i < month - 2; i++) {
days += monthDays[i];
}
if (month > 2) {
days += 1; // 闰年二月有29天
}
days += day;
return days;
}
int main() {
int year, month, day;
printf("请输入日期(年 月 日,用空格分隔):");
scanf("%d %d %d", &year, &month, &day);
// 计算从公元1年1月1日到该日期前一年的年末总共有多少天
int daysBeforeLastYear = daysBeforeYear(year, month, day);
if (isLeapYear(year - 1)) {
daysBeforeLastYear += 366;
} else {
daysBeforeLastYear += 365;
}
printf("从公元1年1月1日到该日期前一年的年末总共有 %d 天\n", daysBeforeLastYear);
// 计算到该日期前一个月的月末总共有多少天
int daysBeforeLastMonth = daysBeforeMonth(year, month, day);
printf("到该日期前一个月的月末总共有 %d 天\n", daysBeforeLastMonth - 1);
// 计算到这一天总共有多少天
int leap = isLeapYear(year); // 是否为闰年
int monthDays[] = {31, 28 + leap, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每个月的天数
int days = 0;
for (int i = 0; i < month - 1; i++) {
days += monthDays[i];
}
days += day;
printf("到这一天总共有 %d 天\n", days - 1);
// 计算这一天是星期几
int daysSinceMonday = (daysBeforeLastYear + days) % 7; // 从星期一开始计算
char weekdays[][10] = {"Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"};
printf("这一天是 %s\n", weekdays[daysSinceMonday]);
return 0;
}
```
这个程序可以实现输入任意一个日期的年、月、日的值,求出从公元1年1月1日到该日期前一年的年末总共有多少天,到该日期前一个月的月末总共有多少天,到这一天总共有多少天,并求出这一天是星期几。
6-4 日期类 - 1. 判断闰年 分数 10 全屏浏览题目 切换布局 作者 李祥 单位 湖北经济学院 请编写函数,判断闰年。 函数原型 // 判断闰年 bool IsLeapYear(int year); 说明:参数 year 为年数。若 year 是闰年,则函数值为true,否则为false。 按照现行历法(格里高利历):四年一闰,百年不闰,四百年再闰。即:年数是 4 的倍数且不是 100 的倍数,或者年数是 400 的倍数,则为闰年,否则为平年。 注:为简化函数设计,作以下限制 年数一律为正整数(即不考虑公元前的情况); 不考虑历法演变过程(即假定从一开始就一直使用现行的历法)。 请按下列步骤,以工程项目的形式来组织程序。 创建文件夹“日期类”,然后将日期类的所有文件都保存到该文件夹中。 进入 Dev-C++,创建 C++ 项目“Project”,保存项目文件 Project.dev。 在项目中创建头文件 MyDate.h 和源程序文件 MyDate.cpp。 在头文件 MyDate.h 中声明日期类。 MyDate.h #ifndef _MyDate_h_ #define _MyDate_h_ // 定义日期类 class MYDATE { public: // 判断闰年 static bool IsLeapYear(int year); }; #endif 在程序文件 MyDate.cpp 中编写函数。 MyDate.cpp #include <iostream> #include <iomanip> using namespace std; #include "MyDate.h" /* 你提交的代码将被嵌在这里 */ 在程序文件 main.cpp 中编写主函数进行测试。 main.cpp #include <iostream> using namespace std; #include "MyDate.h" int main() { int y; cin >> y; if (MYDATE::IsLeapYear(y)) { cout << "闰年\n"; } else { cout << "平年\n"; } return 0; } 输入样例1 2008 输出样例1 闰年 输入样例2 2018 输出样例2 平年 代码长度限制 16 KB 时间限制 400 ms 内存限制 64 MB
MyDate.h:
```cpp
#ifndef _MyDate_h_
#define _MyDate_h_
class MYDATE {
public:
static bool IsLeapYear(int year);
};
#endif
```
MyDate.cpp:
```cpp
#include "MyDate.h"
bool MYDATE::IsLeapYear(int year)
{
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0)
return true;
else
return false;
}
```
main.cpp:
```cpp
#include <iostream>
#include "MyDate.h"
using namespace std;
int main()
{
int y;
cin >> y;
if (MYDATE::IsLeapYear(y))
{
cout << "闰年\n";
}
else
{
cout << "平年\n";
}
return 0;
}
```
阅读全文
相关推荐
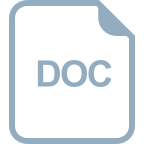
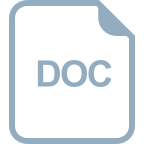
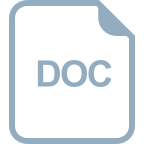
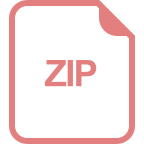
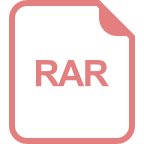
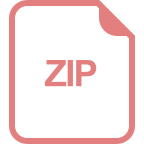
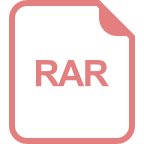
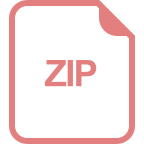
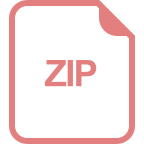
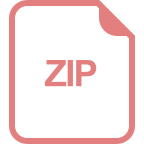
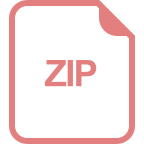
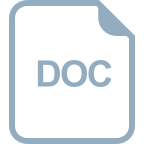
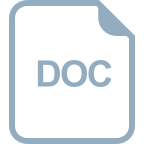
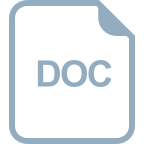
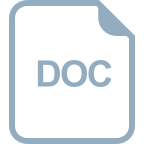
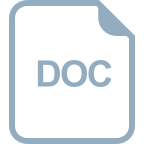
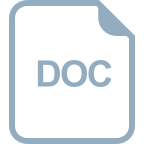