DWA算法如何避障?
时间: 2023-12-23 12:27:58 浏览: 44
DWA算法(Dynamic Window Approach)是一种用于机器人路径规划和避障的算法。它通过考虑机器人的动态窗口,即机器人在给定时间内可能到达的位置和速度范围,来选择最佳的速度和转向角度,以避免障碍物。
DWA算法的避障过程可以通过以下步骤来实现:
1. 获取机器人当前位置和速度信息。
2. 根据机器人的动态窗口,生成一系列可能的速度和转向角度组合。
3. 对于每个速度和转向角度组合,模拟机器人在给定时间内的运动轨迹。
4. 对于每个运动轨迹,计算与障碍物的距离,并评估其与目标位置的距离。
5. 根据评估结果,选择具有最小距离和最小目标距离的速度和转向角度组合作为最佳的运动策略。
6. 将最佳的速度和转向角度应用于机器人,使其避开障碍物并朝向目标位置移动。
下面是一个简单的示例代码,演示了如何使用DWA算法进行避障:
```python
import math
def dwa_algorithm(current_position, current_velocity, obstacles, target_position):
# 定义机器人的动态窗口参数
max_speed = 1.0 # 最大速度
max_yaw_rate = 40.0 * math.pi / 180.0 # 最大转向角速度
max_acceleration = 0.2 # 最大加速度
max_yaw_acceleration = 40.0 * math.pi / 180.0 # 最大转向角加速度
dt = 0.1 # 时间间隔
predict_time = 3.0 # 预测时间
# 生成速度和转向角度组合
velocities = [i * max_speed / 10.0 for i in range(11)]
yaw_rates = [i * max_yaw_rate / 10.0 for i in range(11)]
# 初始化最佳速度和转向角度
best_velocity = 0.0
best_yaw_rate = 0.0
min_distance = float('inf')
# 遍历速度和转向角度组合
for velocity in velocities:
for yaw_rate in yaw_rates:
# 模拟机器人的运动轨迹
x = current_position[0]
y = current_position[1]
theta = current_position[2]
v = velocity
yaw = theta
distance = 0.0
for t in range(int(predict_time / dt)):
# 更新机器人的位置和速度
x += v * math.cos(yaw) * dt
y += v * math.sin(yaw) * dt
yaw += yaw_rate * dt
distance += v * dt
# 计算与障碍物的距离
for obstacle in obstacles:
dx = x - obstacle[0]
dy = y - obstacle[1]
d = math.sqrt(dx**2 + dy**2)
if d < obstacle[2]:
distance += float('inf')
# 计算与目标位置的距离
dx = x - target_position[0]
dy = y - target_position[1]
target_distance = math.sqrt(dx**2 + dy**2)
# 更新最佳速度和转向角度
if distance < min_distance:
min_distance = distance
best_velocity = velocity
best_yaw_rate = yaw_rate
return best_velocity, best_yaw_rate
# 示例使用
current_position = [0.0, 0.0, 0.0]
current_velocity = 0.0
obstacles = [[1.0, 1.0, 0.5], [2.0, 2.0, 0.5]]
target_position = [3.0, 3.0]
best_velocity, best_yaw_rate = dwa_algorithm(current_position, current_velocity, obstacles, target_position)
print("Best velocity: ", best_velocity)
print("Best yaw rate: ", best_yaw_rate)
```
相关推荐
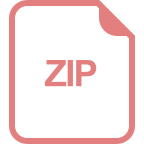
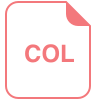













