写出DWA避障算法Python实现
时间: 2023-05-28 17:01:20 浏览: 318
由于DWA算法较为复杂,需要多个函数的配合,下面是一个简化版的Python实现,仅供参考。
```python
import math
# 声明常量
MAX_SPEED = 5.0 # 最大速度
MAX_ROTATION_SPEED = 0.5 # 最大旋转速度
VELOCITY_RESOLUTION = 0.1 # 速度分辨率
ROTATION_SPEED_RESOLUTION = 0.05 # 旋转速度分辨率
OBSTACLE_THRESHOLD = 0.5 # 障碍物距离阈值
STEP_SIZE = 0.1 # 步长
ROBOT_RADIUS = 0.2 # 机器人半径
def get_obstacle_distance(x, y, theta, obstacles):
"""计算机器人到最近障碍物的距离"""
min_distance = float('inf')
for obstacle in obstacles:
obstacle_x, obstacle_y = obstacle
d = math.sqrt(pow(obstacle_x - x, 2) + pow(obstacle_y - y, 2))
if d < min_distance:
min_distance = d
return min_distance
def get_goal_distance(x, y, goal):
"""计算机器人到目标点的距离"""
goal_x, goal_y = goal
return math.sqrt(pow(goal_x - x, 2) + pow(goal_y - y, 2))
def get_allowable_velocities(x, y, theta, obstacles, goal):
"""计算可行的速度"""
allowable_velocities = []
for v in range(int(MAX_SPEED / VELOCITY_RESOLUTION)):
v *= VELOCITY_RESOLUTION
for w in range(int(MAX_ROTATION_SPEED / ROTATION_SPEED_RESOLUTION)):
w -= int(MAX_ROTATION_SPEED / ROTATION_SPEED_RESOLUTION) / 2 # 使旋转速度为0时也能考虑
w *= ROTATION_SPEED_RESOLUTION
xt, yt, thetat = simulate_movement(x, y, theta, v, w) # 模拟运动
obstacle_distance = get_obstacle_distance(xt, yt, thetat, obstacles)
goal_distance = get_goal_distance(xt, yt, goal)
if obstacle_distance > OBSTACLE_THRESHOLD and goal_distance < obstacle_distance:
allowable_velocities.append((v, w))
return allowable_velocities
def simulate_movement(x, y, theta, v, w):
"""模拟运动"""
xt = x + v * math.cos(theta) * STEP_SIZE
yt = y + v * math.sin(theta) * STEP_SIZE
thetat = theta + w * STEP_SIZE
return xt, yt, thetat
def evaluate_controls(x, y, theta, obstacles, goal):
"""计算每个速度的cost,并选择代价最小的"""
allowable_velocities = get_allowable_velocities(x, y, theta, obstacles, goal)
if not allowable_velocities:
return None
allowable_velocities.sort(key=lambda velocity: velocity[1]) # 优先选择小的旋转速度
min_cost = float('inf')
best_velocity = None
for v, w in allowable_velocities:
xt, yt, thetat = simulate_movement(x, y, theta, v, w) # 模拟运动
obstacle_distance = get_obstacle_distance(xt, yt, thetat, obstacles)
goal_distance = get_goal_distance(xt, yt, goal)
cost = 1.5 * obstacle_distance + goal_distance # 代价函数
if cost < min_cost:
min_cost = cost
best_velocity = (v, w)
return best_velocity
def move(x, y, theta, obstacles, goal):
"""执行移动"""
control = evaluate_controls(x, y, theta, obstacles, goal)
if control:
v, w = control
x, y, theta = simulate_movement(x, y, theta, v, w)
return x, y, theta
```
这是一份简单实现,可能存在一些问题,需要自行进行测试和改进。完整的DWA算法实现中,一般还会包括对机器人姿态的处理、路径规划算法的配合等部分。
相关推荐
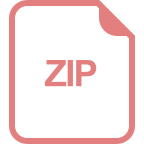
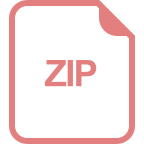














