数据结构BF完整代码实现,C语言版,用串的定长顺序结构
时间: 2024-11-25 15:16:03 浏览: 1
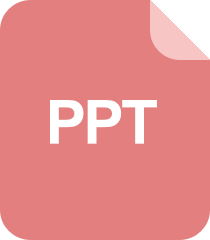
其算法段为-数据结构C语言版教材讲义
Bloom Filter是一种空间效率很高的概率型数据结构,用于快速判断一个元素是否在一个集合中。它通过哈希函数将元素映射到固定大小的位数组上,如果所有哈希函数的结果对应位置都是"1",则认为该元素很可能属于集合;如果有任何一个结果是"0",就认为该元素肯定不在集合中。
以下是C语言中简单实现的一个Bloom Filter的示例,采用定长顺序存储:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define SIZE 1000 // 哈希表大小
#define COUNT 5 // 使用的哈希函数数量
typedef unsigned char bit_t;
bit_t bloom_filter[SIZE]; // 定义位数组
// 哈希函数,这里仅作示例,实际应用需要更好的散列算法
unsigned hash1(char* str) {
return (unsigned)str[0];
}
unsigned hash2(char* str) {
return (unsigned)(str[1] - 'a');
}
void add_to_bloom_filter(const char* item) {
for (int i = 0; i < COUNT; ++i) {
int index = hash1(item) % SIZE;
bloom_filter[index] |= 1 << (hash2(item) % SIZE);
}
}
bool check_in_bloom_filter(const char* item) {
for (int i = 0; i < COUNT; ++i) {
int index1 = hash1(item) % SIZE;
int index2 = hash2(item) % SIZE;
if (!(bloom_filter[index1] & (1 << index2))) {
return false; // 如果有一个位是0,直接返回false
}
}
return true; // 全部匹配,则返回true
}
int main() {
const char* items[] = {"apple", "banana", "cherry", "date"};
for (const char* item : items) {
add_to_bloom_filter(item);
}
// 检查是否存在
printf("Is 'orange' in the filter? %s\n",
check_in_bloom_filter("orange") ? "Yes" : "No");
printf("Is 'peach' in the filter? %s\n",
check_in_bloom_filter("peach") ? "Yes"
阅读全文
相关推荐
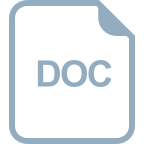
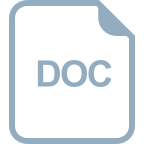

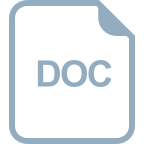
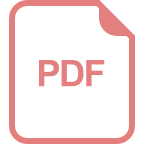
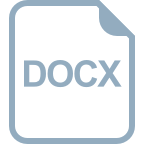
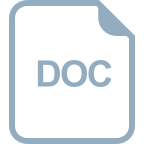
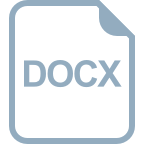
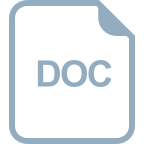
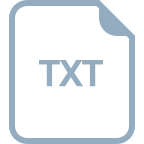
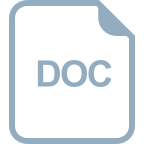
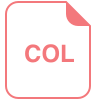
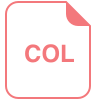
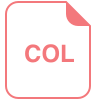
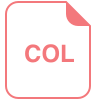
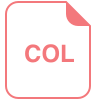
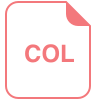
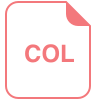