如何用opencvc++和Visual Studio 2022将图片分成等价的四分
时间: 2024-04-18 17:26:20 浏览: 152
要使用OpenCV C++和Visual Studio 2022将图像分成等大小的四个部分,可以按照以下步骤进行操作:
1. 创建一个新的Visual Studio 2022项目,并将其设置为C++项目。
2. 下载并安装OpenCV库,确保已正确配置OpenCV库。
3. 在代码文件中包含必要的头文件:
```cpp
#include <opencv2/opencv.hpp>
```
4. 加载图像:
```cpp
cv::Mat image = cv::imread("your_image.jpg");
```
5. 获取图像的尺寸:
```cpp
int height = image.rows;
int width = image.cols;
```
6. 计算每个子图像的宽度和高度:
```cpp
int sub_width = width / 2;
int sub_height = height / 2;
```
7. 分割图像并保存每个子图像:
```cpp
// 左上角子图像
cv::Mat top_left = image(cv::Rect(0, 0, sub_width, sub_height));
cv::imwrite("top_left.jpg", top_left);
// 右上角子图像
cv::Mat top_right = image(cv::Rect(sub_width, 0, sub_width, sub_height));
cv::imwrite("top_right.jpg", top_right);
// 左下角子图像
cv::Mat bottom_left = image(cv::Rect(0, sub_height, sub_width, sub_height));
cv::imwrite("bottom_left.jpg", bottom_left);
// 右下角子图像
cv::Mat bottom_right = image(cv::Rect(sub_width, sub_height, sub_width, sub_height));
cv::imwrite("bottom_right.jpg", bottom_right);
```
这样就可以将原始图像分成等大小的四个子图像,并将每个子图像保存为独立的文件。
请确保在项目中正确配置了OpenCV库,并且已将OpenCV头文件和库文件的路径添加到项目属性中。
阅读全文
相关推荐
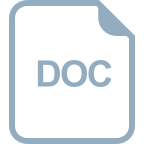
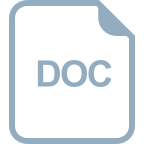
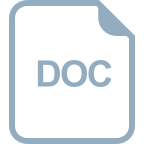


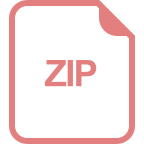
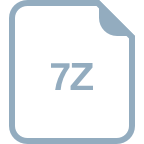
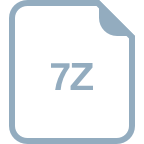
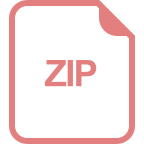
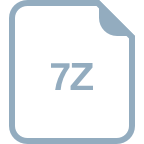
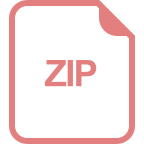
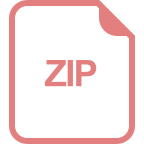
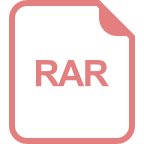
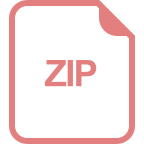
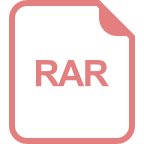


