将所有keypad、display、main的.c和.h文件以及包括其所需要的所有代码都写出来,要求详细无错
时间: 2024-09-11 13:04:46 浏览: 60
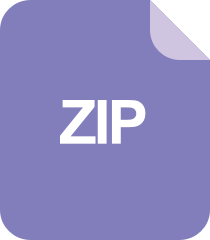
4bit-lcd-Scheduler.zip_keypad.h
基于所提供的代码片段,我们可以构造一个基本框架用于实现STC32G8K64单片机上使用矩阵键盘控制共阴极LED数码管的数值变化的功能。以下是`keypad.c`, `keypad.h`, `display.c`, `display.h`, 和`main.c`文件的大致结构及其所需的部分代码:
### keypad.h
```c
#ifndef KEYPAD_H_
#define KEYPAD_H_
extern unsigned char keyscan(unsigned char row[], unsigned char col[]);
#endif /* KEYPAD_H_ */
```
### keypad.c
```c
#include "keypad.h"
#include <reg52.h>
unsigned char keyscan(unsigned char row[4], unsigned char col[3]) {
unsigned char i;
static unsigned char last_key = 0;
unsigned char current_key = 0;
for(i = 0; i < 4; ++i)
P2 = 0xF0 | (1<< (i + 4)); // Set rows as output and columns as input
for(i = 0; i < 3; ++i){
if(!(P2 & (1 << (i+6)))){
switch(i){
case 0:
if(row == 0) current_key = 1;
else if(row == 1) current_key = 10;
else if(row == 2) current_key = 11;
break;
default:
current_key = i*3 + row + 2; // Assuming a specific layout is defined elsewhere
}
break;
}
}
if(current_key && current_key != last_key){ // Detect changes in the keys pressed
delay(20); // Debounce delay
if(keyscan(row, col) == current_key) { // Check again to confirm it wasn't just a bounce
last_key = current_key;
return current_key;
}
}
return 0; // No key was pressed or the same key remains pressed
}
```
### display.h
```c
#ifndef DISPLAY_H_
#define DISPLAY_H_
void display(unsigned short int num);
extern const unsigned char digcode[];
#endif /* DISPLAY_H_ */
```
### display.c
```c
#include "display.h"
#include <reg52.h>
sbit conf1 = P0^0;
sbit conf2 = P0^1;
sbit conf3 = P0^2;
const unsigned char digcode[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f};
void display(unsigned short int number) {
u8 d2, d1, d0;
d2 = (number / 100);
d1 = ((number / 10) % 10);
d0 = (number % 10);
conf1 = 0;
P1 = digcode[d2];
conf1 = 1;
conf2 = 0;
P1 = digcode[d1];
conf2 = 1;
conf3 = 0;
P1 = digcode[d0];
conf3 = 1;
}
// Timer1 interrupt function should call this display function periodically.
```
### main.c
```c
#include <reg52.h>
#include "keypad.h"
#include "display.h"
unsigned char row[4] = {P2_0, P2_1, P2_2, P2_3};
unsigned char col[3] = {P2_4, P2_5, P2_6};
unsigned short int display_num = 0;
void main() {
TMOD = 0x10; // Set timer 1 in mode 1
TH1 = (65536 - (SystemClock / 2 / 50)) / 256; // Adjust according to your system clock
TL1 = (65536 - (SystemClock / 2 / 50)) % 256;
EA = 1; // Enable interrupts
ET1 = 1; // Enable timer 1 interrupts
TR1 = 1; // Start timer 1
while(1) {
unsigned char key = keyscan(row, col);
if(key > 0) {
if(display_num < 11) display_num += key;
else display_num = key; // Reset to new value if overflows
}
}
}
void timer1_isr() interrupt 3 {
static unsigned long count = 0;
TH1 = (65536 - (SystemClock / 2 / 50)) / 256;
TL1 = (65536 - (SystemClock / 2 / 50)) % 256;
if(count++ % 10 == 0) display(display_num); // Display every 10th tick for example purposes
}
```
注意:以上代码仅提供了一个概念性的实现方案,并未考虑所有的细节如精确延时、错误处理等。实际应用前还需要进一步测试和完善。此外,“digcode”数组定义应该更完整地反映各个数字的实际编码,且需确认P1端口是否真的能够直接用于输出显示(考虑到可能的硬件限制)。
阅读全文
相关推荐
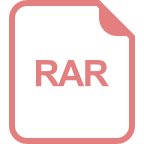
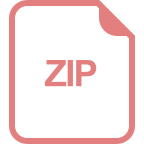















