public static Object copyProperties(Object source, Object target, String... ignoreProperties) { if (source == null) { return target; } BeanUtils.copyProperties(source, target, ignoreProperties); return target; }
时间: 2024-04-17 15:29:00 浏览: 41
这是一个用于属性拷贝的静态方法。它接受三个参数:源对象(source)、目标对象(target)和可变参数(ignoreProperties)。
首先,它会检查源对象是否为null,如果是,则直接返回目标对象。
然后,它使用BeanUtils类的copyProperties方法将源对象的属性拷贝到目标对象中。通过传入的ignoreProperties参数,可以指定需要忽略的属性,这些属性在拷贝过程中将被跳过。
最后,它返回目标对象。
这个方法可以用于将一个对象的属性值拷贝到另一个对象中,方便在不同对象之间进行属性赋值操作。
相关问题
BeanUtils.copyProperties忽略null
BeanUtils.copyProperties方法可以用于对象之间的属性拷贝,但默认情况下它会将源对象的所有属性值拷贝到目标对象中,包括null值。如果需要忽略源对象中的null值属性,可以使用BeanUtils.copyProperties方法的第三个参数,即属性过滤器。
属性过滤器是一个实现了org.springframework.be.BeanUtils.CopyablePropertyFilter接口的对象,它可以控制哪些属性需要被拷贝。在属性过滤器的accept方法中,可以根据属性值是否为null来决定是否拷贝该属性。
以下是一个示例代码,演示了如何使用BeanUtils.copyProperties方法忽略null值:
```java
import org.springframework.beans.BeanUtils;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.BeanWrapperImpl;
import org.springframework.beans.CopyablePropertyFilter;
public class CopyPropertiesExample {
public static void main(String[] args) {
SourceObject source = new SourceObject();
source.setName("John");
source.setAge(25);
source.setAddress(null);
TargetObject target = new TargetObject();
BeanUtils.copyProperties(source, target, (sourceValue, targetValue, targetPropertyDescriptor) -> {
// 忽略源对象中的null值属性
return sourceValue != null;
});
System.out.println(target.getName()); // 输出:John
System.out.println(target.getAge()); // 输出:25
System.out.println(target.getAddress()); // 输出:null
}
}
class SourceObject {
private String name;
private int age;
private String address;
// 省略getter和setter方法
}
class TargetObject {
private String name;
private int age;
private String address;
// 省略getter和setter方法
}
```
在上述示例中,我们创建了一个SourceObject对象,并设置了name、age和address属性,其中address属性的值为null。然后我们创建了一个TargetObject对象,并使用BeanUtils.copyProperties方法将SourceObject的属性拷贝到TargetObject中,通过属性过滤器忽略了源对象中的null值属性。最后我们输出了TargetObject对象的属性值,可以看到address属性的值为null。
BeanUtils.copyProperties忽略null值
可以使用BeanMapper或者在BeanUtils.copyProperties中传入一个自定义的属性过滤器来忽略null值。以下是两种方法的示例代码:
1. 使用BeanMapper忽略null值
```python
import net.sf.cglib.beans.BeanCopier;
import net.sf.cglib.core.Converter;
public class BeanMapper {
private static final Map<String, BeanCopier> BEAN_COPIERS = new ConcurrentHashMap<>();
public static void copyProperties(Object source, Object target) {
String key = source.getClass().toString() + target.getClass().toString();
BeanCopier copier = null;
if (!BEAN_COPIERS.containsKey(key)) {
copier = BeanCopier.create(source.getClass(), target.getClass(), true);
BEAN_COPIERS.put(key, copier);
} else {
copier = BEAN_COPIERS.get(key);
}
copier.copy(source, target, new Converter() {
@Override
public Object convert(Object value, Class target, Object context) {
if (value == null) {
if (target == String.class) {
return "";
} else if (target == Integer.class) {
return 0;
} else if (target == Long.class) {
return 0L;
} else if (target == Double.class) {
return 0.0;
} else if (target == Float.class) {
return 0.0f;
} else if (target == Boolean.class) {
return false;
} else {
return null;
}
} else {
return value;
}
}
});
}
}
```
使用示例:
```python
Source source = new Source();
source.setName("test");
source.setAge(18);
source.setAddress(null);
Target target = new Target();
BeanMapper.copyProperties(source, target);
System.out.println(target.getName()); // 输出:test
System.out.println(target.getAge()); // 输出:18
System.out.println(target.getAddress()); // 输出:null
```
2. 在BeanUtils.copyProperties中传入自定义属性过滤器忽略null值
```python
import org.springframework.beans.BeanUtils;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.BeanWrapperImpl;
import java.beans.PropertyDescriptor;
import java.util.HashSet;
import java.util.Set;
public class CopyUtils {
public static void copyPropertiesIgnoreNull(Object source, Object target) {
BeanUtils.copyProperties(source, target, getNullPropertyNames(source));
}
private static String[] getNullPropertyNames(Object source) {
final BeanWrapper src = new BeanWrapperImpl(source);
PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set<String> emptyNames = new HashSet<>();
for (PropertyDescriptor pd : pds) {
Object srcValue = src.getPropertyValue(pd.getName());
if (srcValue == null) {
emptyNames.add(pd.getName());
}
}
String[] result = new String[emptyNames.size()];
return emptyNames.toArray(result);
}
}
```
使用示例:
```python
Source source = new Source();
source.setName("test");
source.setAge(18);
source.setAddress(null);
Target target = new Target();
CopyUtils.copyPropertiesIgnoreNull(source, target);
System.out.println(target.getName()); // 输出:test
System.out.println(target.getAge()); // 输出:18
System.out.println(target.getAddress()); // 输出:null
```
阅读全文
相关推荐
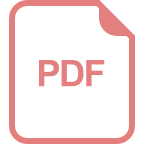
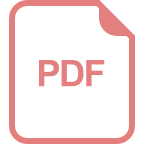
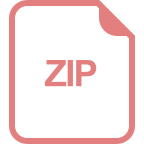
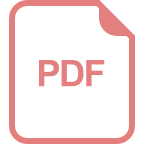
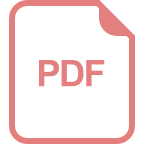





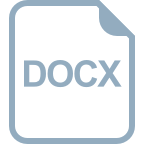
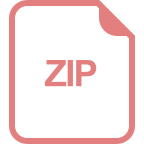