yolo8+ c++opencv
时间: 2023-08-31 14:02:21 浏览: 252
YOLO(You Only Look Once)是一种实时目标检测算法,它使用深度学习模型在一次前向传递中同时对图像进行了对象检测和分类。YOLO算法因其高效性和准确性而受到广泛关注。
YOLO算法中的数字8可能是指YOLOv3(YOLO的第三个版本),这是最常用和流行的YOLO版本。YOLOv3采用了Darknet-53神经网络作为基础架构,并在该基础上使用了多个尺度的特征图来检测不同大小的对象。此外,它还通过使用卷积层和跳跃连接来提高特征的表示能力和检测的准确性。
C语言是一种广泛使用的编程语言,可以用于实现YOLO算法。C语言的高效性和与硬件底层的紧密结合使得它成为实时目标检测算法的理想选择。开发者可以使用C语言编写YOLO算法的推理代码,以便在不同平台上进行高效的目标检测任务。
OpenCV是一种开源的计算机视觉库,提供了许多用于图像处理和计算机视觉任务的函数和工具。通过结合OpenCV和YOLO算法,开发者可以方便地实现实时目标检测,包括加载YOLO模型、对图像或视频进行对象检测、绘制边界框以及执行对象分类等任务。
综上所述,YOLO算法是一种实时目标检测算法,YOLOv3是其中最常用和流行的版本。C语言可以用于实现YOLO算法,而OpenCV是一种计算机视觉库,可以方便地实现与YOLO算法相关的图像处理和目标检测任务。
相关问题
opencv+c++YOLO
YOLO (You Only Look Once) is an object detection algorithm that uses deep neural networks to detect objects in real-time. OpenCV is a popular computer vision library that provides various tools and functions for image processing and computer vision tasks.
To use YOLO with OpenCV in C++, you need to follow these steps:
1. Download the YOLOv3 weights and configuration files from the official website.
2. Load the model using OpenCV's dnn module.
3. Read the input image and preprocess it.
4. Pass the image through the model to get the output.
5. Postprocess the output to get the object detection results.
6. Draw bounding boxes around the detected objects and display the output image.
Here's a sample code that demonstrates how to use YOLO with OpenCV in C++:
```
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main()
{
// Load YOLOv3 model
String modelWeights = "yolov3.weights";
String modelConfiguration = "yolov3.cfg";
dnn::Net net = dnn::readNetFromDarknet(modelConfiguration, modelWeights);
// Read input image
Mat image = imread("input.jpg");
// Preprocess input image
Mat blob = dnn::blobFromImage(image, 1/255.0, Size(416, 416), Scalar(0,0,0), true, false);
// Set input for the network
net.setInput(blob);
// Get output from the network
vector<Mat> outs;
net.forward(outs, net.getUnconnectedOutLayersNames());
// Postprocess the output
float confThreshold = 0.5;
vector<int> classIds;
vector<float> confidences;
vector<Rect> boxes;
for (size_t i = 0; i < outs.size(); ++i)
{
// Extract information from the output
Mat output = outs[i];
for (int j = 0; j < output.rows; ++j)
{
Mat scores = output.row(j).colRange(5, output.cols);
Point classId;
double confidence;
minMaxLoc(scores, 0, &confidence, 0, &classId);
if (confidence > confThreshold)
{
// Get bounding box coordinates
int centerX = static_cast<int>(output.at<float>(j, 0) * image.cols);
int centerY = static_cast<int>(output.at<float>(j, 1) * image.rows);
int width = static_cast<int>(output.at<float>(j, 2) * image.cols);
int height = static_cast<int>(output.at<float>(j, 3) * image.rows);
int left = centerX - width / 2;
int top = centerY - height / 2;
// Save detection results
classIds.push_back(classId.x);
confidences.push_back(static_cast<float>(confidence));
boxes.push_back(Rect(left, top, width, height));
}
}
}
// Draw bounding boxes around the detected objects
vector<String> classNames = {"person", "car", "bus", "truck"};
vector<Scalar> colors = {Scalar(0, 0, 255), Scalar(255, 0, 0), Scalar(0, 255, 0), Scalar(255, 255, 0)};
for (size_t i = 0; i < boxes.size(); ++i)
{
rectangle(image, boxes[i], colors[classIds[i]], 2);
String label = format("%.2f", confidences[i]);
label = classNames[classIds[i]] + ":" + label;
int baseLine;
Size labelSize = getTextSize(label, FONT_HERSHEY_SIMPLEX, 0.5, 1, &baseLine);
rectangle(image, Point(boxes[i].x, boxes[i].y - labelSize.height - baseLine), Point(boxes[i].x + labelSize.width, boxes[i].y), colors[classIds[i]], FILLED);
putText(image, label, Point(boxes[i].x, boxes[i].y - baseLine), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(255,255,255));
}
// Display the output image
imshow("YOLO Object Detection", image);
waitKey(0);
return 0;
}
```
Note that this sample code is for YOLOv3 and may need to be modified for other versions of YOLO. Also, make sure to download the correct weights and configuration files for the version you are using.
OpenCV:YOLO目标检测 c++
YOLO(You Only Look Once)是一种流行的目标检测算法,它能够快速准确地在图像中识别和定位多个对象。YOLO算法将目标检测任务视为一个回归问题,通过一个单一的神经网络直接从图像像素到类别概率和边界框坐标的映射。
在C++中使用OpenCV实现YOLO目标检测通常涉及以下步骤:
1. 加载YOLO模型:首先需要从预训练的YOLO模型文件中加载网络,这些文件包括配置文件(.cfg),预训练权重文件(.weights)和类别名称文件(.names)。
2. 图像预处理:在进行目标检测之前,需要对输入图像进行预处理,比如缩放到网络输入尺寸,归一化等。
3. 进行目标检测:将预处理后的图像输入到YOLO网络中,网络会输出包含多个边界框和对应置信度的预测结果。
4. 筛选和输出结果:根据阈值筛选出置信度高的边界框,并将它们转换为原始图像尺寸上的位置坐标。输出这些边界框和对应的类别标签。
在OpenCV中,YOLO模型通常使用DNN模块来加载和运行。可以使用`cv::dnn::readNetFromDarknet`函数来加载配置和权重文件,并使用`cv::dnn::Net::forward`函数来执行前向传播获取检测结果。
阅读全文
相关推荐
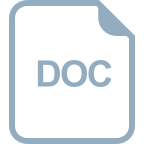
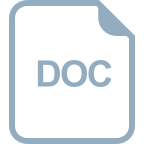
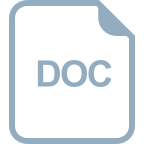
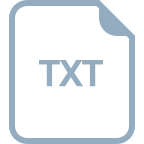
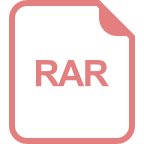
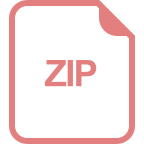
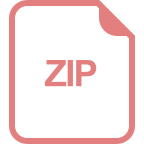
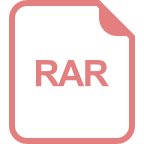
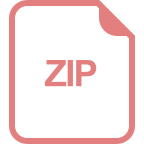
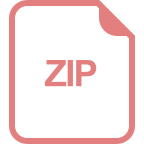
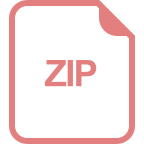
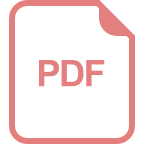
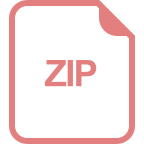
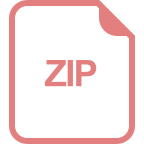
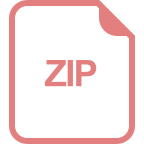
