在C#中,从我发布网站的服务器中复制文件到另一台服务器的指定文件夹内,代码事例
时间: 2024-09-09 21:11:57 浏览: 30
在C#中,你可以使用`System.IO`命名空间下的`File.Copy()`方法或者`Directory.CreateDirectory()`和`File.Move()`方法结合来实现文件的复制操作。以下是一个简单的示例,展示了如何将文件从源服务器复制到目标服务器的指定目录:
```csharp
using System;
using System.IO;
public static void CopyFileFromServer(string sourceFilePath, string destinationServerAddress, string destinationFolder)
{
// 源服务器路径加上文件名
string sourceServerPath = "http://" + sourceFilePath;
try
{
// 创建目标目录,如果不存在
if (!Directory.Exists(destinationServerAddress + destinationFolder))
{
Directory.CreateDirectory(destinationServerAddress + destinationFolder);
Console.WriteLine($"创建了目标目录: {destinationServerAddress}{destinationFolder}");
}
// 获取文件内容并复制到新的位置
using (var client = new WebClient())
{
var fileBytes = client.DownloadData(sourceServerPath);
var targetFilePath = destinationServerAddress + destinationFolder + Path.GetFileName(sourceFilePath);
File.WriteAllBytes(targetFilePath, fileBytes);
Console.WriteLine($"成功复制文件到: {targetFilePath}");
}
}
catch (Exception ex)
{
Console.WriteLine($"文件复制过程中发生错误: {ex.Message}");
}
}
// 调用函数,将sourceFilePath替换为你源服务器的文件路径,destinationServerAddress为目标服务器地址,destinationFolder为目标文件夹路径
CopyFileFromServer("your-source-file.txt", "http://your-target-server.com", "/path/to/destination/folder");
```
注意,这个例子假设你有足够的权限访问目标服务器,并且目标服务器支持HTTP访问。如果需要其他协议(如FTP、SFTP等),你可能需要使用相应的库来进行网络操作。
相关推荐
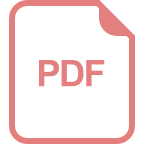
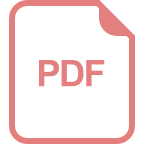
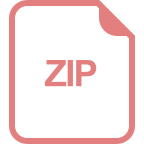














