``` const customerTime = ref(`${now.getFullYear()}-${String(now.getMonth() + 1).padStart(2, '0')}`); ```
时间: 2024-09-20 10:14:14 浏览: 53
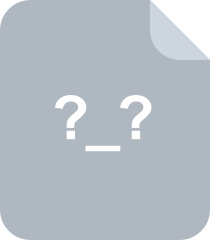
VC++ string_format(const char* format, ...)

这段代码是用 Vue.js 的 `ref` 函数来创建一个名为 `customerTime` 的引用对象,它的目的是存储当前日期的年-月格式。具体解释如下:
1. `const customerTime = ref`: `ref` 是 Vue 中用于数据绑定和响应式管理的一个工具,它返回一个响应式的 JavaScript 值。
2. `${now.getFullYear()}`: `now` 表示获取当前时间(JavaScript 的 `Date.now()` 或者 `new Date().getTime()` 等效),`getFullYear()` 方法返回当前年份。这部分构建了当前年份的部分。
3. `-${String(now.getMonth() + 1)}`: `getMonth()` 返回的是 0 到 11 之间的月份,所以要加 1 得到实际的月份。然后将其转换为字符串,`String()` 函数确保得到的是文本形式的数字,例如 "07"。
4. `.padStart(2, '0')`: 这是一个字符串方法,用于在字符串前面填充指定字符,直到达到指定长度。这里将月份始终填充到两位数,不足两位时前面补零。例如,如果月份是 8,则会变成 "08"。
最终,`customerTime` 将保存一个类似于 "2022-07"(假设现在是 2022 年 7 月)这样的字符串,表示顾客的时间信息。
阅读全文
相关推荐
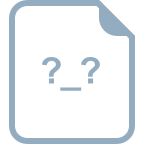
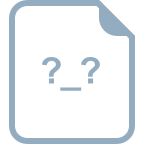



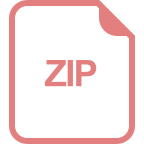
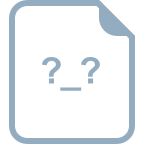
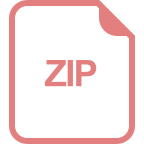
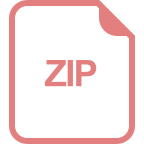
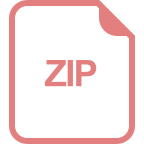
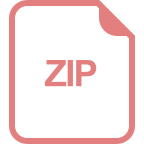
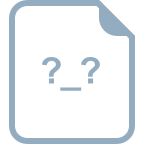
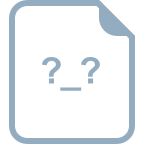
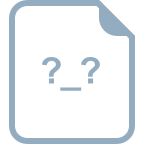
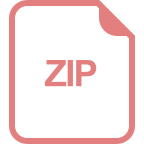
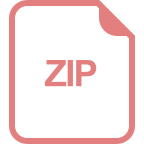
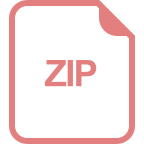
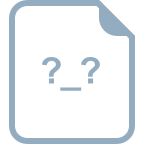