python RGB图像特征提取
时间: 2023-11-06 11:03:43 浏览: 208
对于Python中的RGB图像特征提取,你可以使用各种图像处理库和技术来实现。其中一种常用的方法是使用OpenCV库。以下是一些常见的特征提取技术:
1. 颜色直方图:通过统计图像中每个颜色通道的像素数量来描述图像的颜色分布。你可以使用OpenCV的`cv2.calcHist`函数来计算颜色直方图。
2. 纹理特征:纹理特征描述图像的纹理细节,例如图像中的纹理模式、方向和对比度等。你可以使用GLCM(灰度共生矩阵)或LBP(局部二值模式)等方法来提取纹理特征。
3. 形状特征:形状特征描述了图像中物体的形状和结构。常见的形状特征包括边缘检测、轮廓提取和形状描述子等。
4. SIFT或SURF特征:这些是基于局部特征的方法,可以检测并描述图像中的关键点和局部特征。
你可以使用OpenCV和其他图像处理库来实现这些特征提取技术。具体的代码实现和使用方法可以参考引用和引用中提供的教程和示例代码。
相关问题
python实现GLCM图像特征提取
GLCM(Gray-Level Co-occurrence Matrix)是一种用于图像特征提取的方法,可以用于描述图像中像素灰度值之间的关系。下面是python实现GLCM图像特征提取的步骤:
1. 将图像转换为灰度图像。
2. 根据需要选择距离和角度。常用的距离为1和2,常用的角度为0度、45度、90度和135度。
3. 根据选择的距离和角度计算GLCM矩阵。
4. 根据GLCM矩阵计算出各种统计量,如能量、对比度、均值等。
下面是python代码示例:
```python
import numpy as np
from skimage.feature import greycomatrix, greycoprops
from skimage import io, color
# 读取图像并转换为灰度图像
img = io.imread('image.jpg')
img_gray = color.rgb2gray(img)
# 计算GLCM矩阵
d = 1 # 距离
angles = [0, np.pi/4, np.pi/2, 3*np.pi/4] # 角度
glcm = greycomatrix(img_gray, distances=[d], angles=angles, levels=256, symmetric=True, normed=True)
# 计算GLCM统计量
contrast = greycoprops(glcm, 'contrast')
dissimilarity = greycoprops(glcm, 'dissimilarity')
homogeneity = greycoprops(glcm, 'homogeneity')
energy = greycoprops(glcm, 'energy')
correlation = greycoprops(glcm, 'correlation')
ASM = greycoprops(glcm, 'ASM')
```
其中,`img`为原始图像,`img_gray`为灰度图像,`d`为距离,`angles`为角度,`levels`为灰度级数,`symmetric`为是否对称,`normed`为是否进行归一化。`glcm`为计算得到的GLCM矩阵,`contrast`、`dissimilarity`、`homogeneity`、`energy`、`correlation`和`ASM`为计算得到的GLCM统计量。
python提取rgb图像的三个通道
在Python中,你可以使用PIL(Python Imaging Library)或者其更新版Pillow库来提取RGB图像的三个通道。这两个库提供了方便的功能来处理图像。以下是使用Pillow库的一个简单示例:
```python
from PIL import Image
def extract_rgb_channels(image_path):
# 打开图像文件
img = Image.open(image_path)
# 将图像转换为像素矩阵
pixels = list(img.getdata())
# 分别获取红(R)、绿(G)、蓝(B)通道的像素列表
r_channel = [pixel[0] for pixel in pixels]
g_channel = [pixel[1] for pixel in pixels]
b_channel = [pixel[2] for pixel in pixels]
return r_channel, g_channel, b_channel
# 使用方法
r_channel, g_channel, b_channel = extract_rgb_channels('image.jpg')
```
在这个例子中,`img.getdata()`返回的是一个包含所有像素点的二维列表,每个像素点是一个包含红色、绿色和蓝色分量的元组。通过列表推导式,我们分别提取了这三个通道的值。
阅读全文
相关推荐
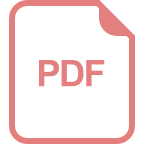
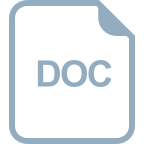
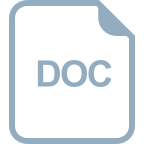
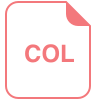
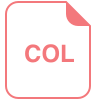
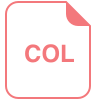
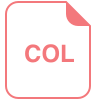
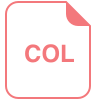





