python 列表写入json
时间: 2024-08-01 10:01:25 浏览: 53
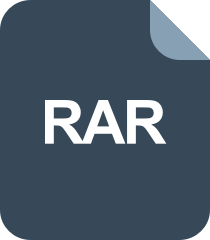
将Python列表内容写入JSON文件并存储的两种方法

在Python中,你可以使用内置的`json`模块将列表数据转换成JSON格式并写入文件。以下是一个简单的例子:
```python
import json
# 假设我们有一个列表
data_list = ["apple", "banana", "cherry"]
# 将列表转换为字典,因为json库默认接收的是字典类型的数据
data_dict = {"fruits": data_list}
# 写入到json文件
with open('fruits.json', 'w') as f:
json.dump(data_dict, f)
阅读全文
相关推荐
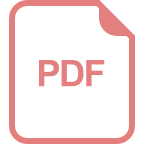
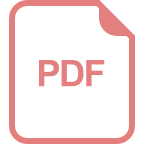













