python中json模块写入json文件常用方法
时间: 2024-03-01 21:48:04 浏览: 114
在Python中,可以使用json模块来读取和写入JSON文件。下面是一些常用的写入JSON文件的方法:
1. 使用json.dump()函数:该函数将Python对象转换为JSON格式,并将其写入文件中。示例代码如下:
```python
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
with open("data.json", "w") as file:
json.dump(data, file)
```
2. 使用json.dumps()函数:该函数将Python对象转换为JSON格式的字符串,然后可以将字符串写入文件。示例代码如下:
```python
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
json_data = json.dumps(data)
with open("data.json", "w") as file:
file.write(json_data)
```
这两种方法都可以将Python对象写入JSON文件中。需要注意的是,使用这些方法写入的JSON文件是以UTF-8编码保存的。
相关问题
python 以json格式写入json文件
在 Python 中,可以使用 `json.dump()` 函数将 JSON 数据写入 JSON 文件。该函数的语法如下:
```python
import json
with open('filename.json', 'w') as f:
json.dump(data, f)
```
其中,`filename.json` 是要写入的 JSON 文件名,`data` 是要写入的 JSON 数据,`f` 是文件对象。使用 `with` 语句可以确保在写入完成后自动关闭文件。
例如,将一个字典对象写入 JSON 文件:
```python
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
with open('data.json', 'w') as f:
json.dump(data, f)
```
这将在当前目录下创建一个名为 `data.json` 的文件,并将 `data` 字典对象以 JSON 格式写入该文件。
如果要将一个列表对象写入 JSON 文件,可以使用 `json.dump()` 函数的第三个参数 `indent` 来设置缩进:
```python
import json
data = ['apple', 'banana', 'orange']
with open('data.json', 'w') as f:
json.dump(data, f, indent=4)
```
这将在当前目录下创建一个名为 `data.json` 的文件,并将 `data` 列表对象以缩进为 4 的 JSON 格式写入该文件。
python创建json文件写入,如何在json文件写入换行
在Python中创建JSON文件并写入换行,可以使用`json.dumps`方法将JSON对象转换为字符串,然后在写入文件时,使用参数`indent`来设置缩进,并且在写入字符串前加上换行符。以下是一个示例代码:
```python
import json
data = {
"name": "John",
"age": 30,
"city": "New York"
}
with open('data.json', 'w') as f:
json.dump(data, f, indent=4)
f.write('\n')
```
在上面的代码中,我们将JSON对象`data`转换为字符串,并使用`json.dump`方法将其写入文件。`indent=4`参数告诉`json.dump`方法在写入文件时使用4个空格进行缩进。最后,我们在写入前使用`f.write('\n')`语句添加一个换行符。
这样,我们就可以在JSON文件中写入换行了。
阅读全文
相关推荐
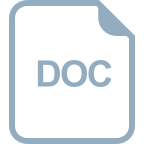
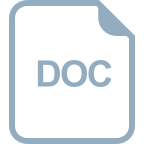
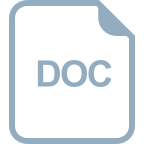
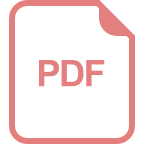
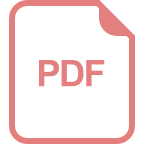











