java headers设置代理
时间: 2024-09-20 14:03:55 浏览: 34
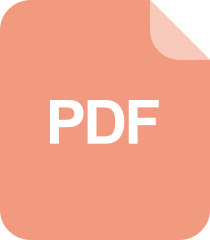
selenium设置proxy、headers的方法(phantomjs、Chrome、Firefox)
在Java中,如果你需要设置HTTP请求头(Headers)并通过代理服务器发送网络请求,通常是在`java.net.HttpURLConnection`或者第三方库如Apache HttpClient中操作。这里是一个例子,展示如何使用`HttpURLConnection`设置代理:
```java
import java.net.HttpURLConnection;
import java.net.Proxy;
import java.net.URL;
public class ProxyExample {
public static void main(String[] args) throws Exception {
URL url = new URL("http://example.com");
Proxy proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress("your-proxy-host", your-proxy-port)); // 替换为你的代理主机和端口
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setUseCaches(false); // 关闭缓存,避免影响代理设置
connection.setRequestProperty("User-Agent", "Mozilla/5.0"); // 添加请求头,根据实际需求可能还需要其他header
connection.setRequestProperty("Accept-Language", "en-US,en;q=0.5"); // 可选,设置请求接受的语言
connection.setConnectTimeout(5000); // 设置连接超时时间
// 通过proxy设置连接
connection.setProxy(proxy);
// 打开连接并执行请求
connection.connect();
// ... 这里处理响应,比如读取输入流
System.out.println(connection.getResponseCode());
connection.disconnect();
}
}
```
同样,如果你使用的是Apache HttpClient,设置会有所不同:
```java
import org.apache.http.client.config.RequestConfig;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class HttpClientProxyExample {
public static void main(String[] args) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
RequestConfig config = RequestConfig.custom()
.setProxy(new HttpHost("your-proxy-host", your-proxy-port))
.build();
HttpGet httpGet = new HttpGet("http://example.com");
httpGet.setHeader("User-Agent", "Mozilla/5.0");
CloseableHttpResponse response = httpClient.execute(httpGet, config);
try {
String responseBody = EntityUtils.toString(response.getEntity(), StandardCharsets.UTF_8);
System.out.println(responseBody);
} finally {
response.close();
httpClient.close();
}
}
}
```
请注意,`your-proxy-host`和`your-proxy-port`应替换为你实际使用的代理服务器的主机名和端口号。同时,确保你的代理支持HTTPS(如果是用于HTTPS请求),或者开启透明代理功能。
阅读全文
相关推荐
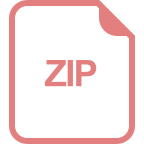
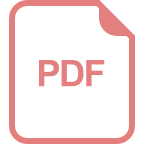
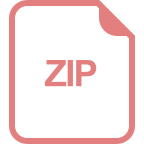
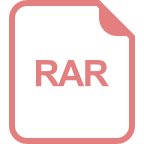
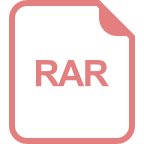
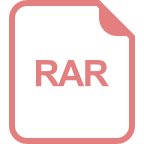
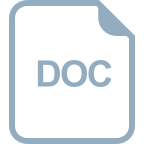
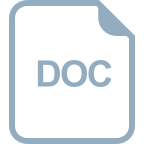
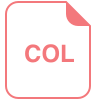
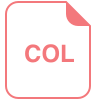






