C语言编写打印月历的程序,打印2024年2月的月历
时间: 2024-10-17 14:13:37 浏览: 67
在C语言中,我们可以利用`<stdio.h>`库中的函数来编写一个简单的程序,打印出指定月份的月历。对于2024年2月,由于闰年的规则,我们需要检查该年是否能被4整除,如果能并且不是100的倍数,或者能被400整除,则为闰年。下面是打印2024年2月月历的一个基本示例:
```c
#include <stdio.h>
#include <time.h>
// 检查是否为闰年
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 打印一个月的日历
void print_calendar(int year, int month) {
const int days_in_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 根据月份计算天数
struct tm calendar[1];
if(is_leap_year(year) && month == 2) {
days_in_month[1] = 29; // 闰年2月有29天
}
calendar[0].tm_mday = 1;
calendar[0].tm_mon = month - 1;
calendar[0].tm_year = year - 1900;
for (int i = 0; i < days_in_month[month - 1]; ++i) {
time_t t = mktime(calendar);
printf("%d ", localtime(&t)->tm_mday); // 显示日期
if ((i + 1) % 7 == 0) { // 每周换行
printf("\n");
}
}
printf("\n");
}
int main() {
int year = 2024;
int month = 2;
print_calendar(year, month);
return 0;
}
```
当你运行这个程序时,它会输出2024年2月的完整月历。注意,这里我们假设用户输入正确的月份,实际应用中可能需要加入错误处理。
阅读全文
相关推荐




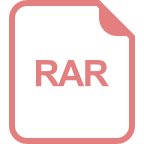



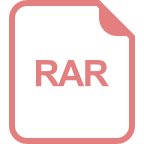