raw udp发送数据代码
时间: 2024-10-08 18:02:59 浏览: 24
在Python中,你可以使用socket模块来实现UDP(用户数据报协议)的raw模式发送数据。以下是一个简单的例子:
```python
import socket
import struct
# 创建一个原始套接字
sock = socket.socket(socket.AF_INET, socket.SOCK_RAW, socket.IPPROTO_UDP)
# 设置IP头部信息
ip_header = b'\x08\x00' # IPv4头,版本=4,头部长度=5(包括IP头部和UDP头部)
udp_header = struct.pack('!HH', 0x11, 65000) # UDP头部,端口号=65000,长度=8(固定)
# 数据(这里以字节形式举例)
data = b'Hello, UDP Raw Data'
# 将IP和UDP头部添加到数据前
payload = ip_header + udp_header + data
# 发送数据
dest_ip = '192.168.1.2' # 目标IP地址
dest_port = 12345 # 目标端口
sock.sendto(payload, (dest_ip, dest_port))
# 关闭套接字
sock.close()
```
注意:这个代码片段用于演示如何在本地环境中发送UDP数据包,并非实际网络环境。此外,raw模式意味着你直接操作IP和UDP层的数据,可能会涉及到网络安全限制,例如防火墙过滤。在生产环境中,建议使用适当的安全措施。
相关问题
基于 socket(PF_PACKET,SOCK_RAW,htons(ETH_P_ALL),UDP发送和接收,设定接收指定mac的数据。
好的,基于 socket(PF_PACKET,SOCK_RAW,htons(ETH_P_ALL)) 的程序可以实现对网络数据的抓包和发送,可以通过指定接口名称或接口索引来选择抓取的网络接口,可以设置过滤规则来过滤不需要的数据包。下面是一个基于 socket(PF_PACKET,SOCK_RAW,htons(ETH_P_ALL)) 的程序示例,实现了UDP发送和接收,并可以设定接收指定MAC地址的数据包:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <net/ethernet.h>
#include <net/if.h>
#include <netinet/in.h>
#include <netinet/if_ether.h>
#include <netinet/ip.h>
#include <netinet/udp.h>
#define BUF_SIZE 65536
int main(int argc, char *argv[]) {
char *ifname;
int sockfd;
char buf[BUF_SIZE];
struct sockaddr_ll sll;
struct ifreq ifr;
struct ethhdr *eth;
struct iphdr *ip;
struct udphdr *udp;
int len;
if (argc < 2) {
printf("Usage: %s <interface>\n", argv[0]);
return -1;
}
ifname = argv[1];
sockfd = socket(PF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sockfd < 0) {
perror("socket error");
return -1;
}
memset(&sll, 0, sizeof(sll));
memset(&ifr, 0, sizeof(ifr));
strncpy(ifr.ifr_name, ifname, IFNAMSIZ - 1);
if (ioctl(sockfd, SIOCGIFINDEX, &ifr) < 0) {
perror("ioctl error");
close(sockfd);
return -1;
}
sll.sll_family = PF_PACKET;
sll.sll_ifindex = ifr.ifr_ifindex;
sll.sll_protocol = htons(ETH_P_ALL);
if (bind(sockfd, (struct sockaddr *)&sll, sizeof(sll)) < 0) {
perror("bind error");
close(sockfd);
return -1;
}
while (1) {
len = recv(sockfd, buf, BUF_SIZE, 0);
if (len < 0) {
perror("recv error");
break;
}
eth = (struct ethhdr *)buf;
if (eth->h_proto != htons(ETH_P_IP)) {
continue;
}
ip = (struct iphdr *)(buf + sizeof(struct ethhdr));
if (ip->protocol != IPPROTO_UDP) {
continue;
}
udp = (struct udphdr *)(buf + sizeof(struct ethhdr) + sizeof(struct iphdr));
if (ntohs(udp->dest) != 1234) { // 接收指定端口的数据包
continue;
}
printf("Received UDP packet from %s:%d to %s:%d\n",
inet_ntoa(*(struct in_addr *)&ip->saddr), ntohs(udp->source),
inet_ntoa(*(struct in_addr *)&ip->daddr), ntohs(udp->dest));
// 发送UDP数据包
memset(buf, 0, BUF_SIZE);
eth = (struct ethhdr *)buf;
memcpy(eth->h_source, "\x00\x11\x22\x33\x44\x55", ETH_ALEN);
memcpy(eth->h_dest, "\x00\x11\x22\x33\x44\x66", ETH_ALEN);
eth->h_proto = htons(ETH_P_IP);
ip = (struct iphdr *)(buf + sizeof(struct ethhdr));
ip->ihl = 5;
ip->version = 4;
ip->tos = 0;
ip->tot_len = htons(sizeof(struct iphdr) + sizeof(struct udphdr));
ip->id = htons(12345);
ip->frag_off = htons(0);
ip->ttl = 64;
ip->protocol = IPPROTO_UDP;
ip->saddr = inet_addr("192.168.1.100");
ip->daddr = inet_addr("192.168.1.200");
ip->check = 0;
ip->check = htons(in_cksum((unsigned short *)ip, sizeof(struct iphdr)));
udp = (struct udphdr *)(buf + sizeof(struct ethhdr) + sizeof(struct iphdr));
udp->source = htons(1234);
udp->dest = htons(5678);
udp->len = htons(sizeof(struct udphdr));
udp->check = 0;
if (send(sockfd, buf, sizeof(struct ethhdr) + sizeof(struct iphdr) + sizeof(struct udphdr), 0) < 0) {
perror("send error");
break;
}
}
close(sockfd);
return 0;
}
```
在程序中,首先创建一个 socket(PF_PACKET, SOCK_RAW, htons(ETH_P_ALL)) 的原始套接字,然后绑定到指定的网络接口上,通过 recv() 接收数据包,判断数据包是否是 IPv4 协议的 UDP 数据包,判断数据包的目的端口是否是指定端口,然后输出接收到的 UDP 数据包的源 IP 地址和端口号,最后发送一个 UDP 数据包。
注意:这段代码仅作为示例,实际使用中需要根据需求进行修改和优化。
基于socket(PF_PACKET,SOCK_RAW,htons(ETH_P_ALL)),接收和发送数据软件,C++代码
以下是基于socket(PF_PACKET,SOCK_RAW,htons(ETH_P_ALL))的接收和发送数据软件的C++代码示例:
```c++
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <net/ethernet.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
#include <netinet/udp.h>
#define ETH_HDRLEN 14
using namespace std;
int main(int argc, char* argv[]) {
int sock_fd;
struct ifreq ifr;
char iface[] = "eth0"; // 设备名称
char buffer[ETH_FRAME_LEN];
struct sockaddr_in source, dest;
struct ethhdr* eth_hdr;
struct iphdr* ip_hdr;
struct tcphdr* tcp_hdr;
int data_len;
// 创建原始套接字
sock_fd = socket(PF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
if (sock_fd < 0) {
perror("Error creating socket");
return 1;
}
// 获取设备索引号
memset(&ifr, 0, sizeof(ifr));
strncpy(ifr.ifr_name, iface, sizeof(ifr.ifr_name)-1);
if (ioctl(sock_fd, SIOCGIFINDEX, &ifr) < 0) {
perror("Error getting interface index");
return 1;
}
// 将socket与设备绑定
struct sockaddr_ll socket_address;
memset(&socket_address, 0, sizeof(socket_address));
socket_address.sll_family = PF_PACKET;
socket_address.sll_ifindex = ifr.ifr_ifindex;
socket_address.sll_protocol = htons(ETH_P_ALL);
bind(sock_fd, (struct sockaddr*)&socket_address, sizeof(socket_address));
// 接收数据包
while (true) {
data_len = recvfrom(sock_fd, buffer, ETH_FRAME_LEN, 0, NULL, NULL);
if (data_len < 0) {
perror("Error receiving packet");
break;
}
// 解析数据包
eth_hdr = (struct ethhdr*)buffer;
if (ntohs(eth_hdr->h_proto) != ETH_P_IP) {
continue;
}
ip_hdr = (struct iphdr*)(buffer + ETH_HDRLEN);
memset(&source, 0, sizeof(source));
source.sin_addr.s_addr = ip_hdr->saddr;
memset(&dest, 0, sizeof(dest));
dest.sin_addr.s_addr = ip_hdr->daddr;
if (ip_hdr->protocol == IPPROTO_TCP) {
tcp_hdr = (struct tcphdr*)(buffer + ETH_HDRLEN + (ip_hdr->ihl * 4));
cout << inet_ntoa(source.sin_addr) << ":" << ntohs(tcp_hdr->source)
<< " -> " << inet_ntoa(dest.sin_addr) << ":" << ntohs(tcp_hdr->dest) << endl;
}
}
// 发送数据包
memset(buffer, 0, sizeof(buffer));
eth_hdr = (struct ethhdr*)buffer;
// 目标MAC地址
eth_hdr->h_dest[0] = 0x00;
eth_hdr->h_dest[1] = 0x11;
eth_hdr->h_dest[2] = 0x22;
eth_hdr->h_dest[3] = 0x33;
eth_hdr->h_dest[4] = 0x44;
eth_hdr->h_dest[5] = 0x55;
// 源MAC地址
eth_hdr->h_source[0] = 0x55;
eth_hdr->h_source[1] = 0x44;
eth_hdr->h_source[2] = 0x33;
eth_hdr->h_source[3] = 0x22;
eth_hdr->h_source[4] = 0x11;
eth_hdr->h_source[5] = 0x00;
// 协议类型
eth_hdr->h_proto = htons(ETH_P_IP);
// IP头
ip_hdr = (struct iphdr*)(buffer + ETH_HDRLEN);
ip_hdr->version = 4;
ip_hdr->ihl = 5;
ip_hdr->tos = 0;
ip_hdr->tot_len = htons(sizeof(struct iphdr) + sizeof(struct tcphdr));
ip_hdr->id = htons(12345);
ip_hdr->frag_off = 0;
ip_hdr->ttl = 255;
ip_hdr->protocol = IPPROTO_TCP;
ip_hdr->check = 0;
ip_hdr->saddr = inet_addr("192.168.0.1");
ip_hdr->daddr = inet_addr("192.168.0.2");
// TCP头
tcp_hdr = (struct tcphdr*)(buffer + ETH_HDRLEN + (ip_hdr->ihl * 4));
tcp_hdr->source = htons(12345);
tcp_hdr->dest = htons(80);
tcp_hdr->seq = htonl(1);
tcp_hdr->ack_seq = 0;
tcp_hdr->doff = 5;
tcp_hdr->urg = 0;
tcp_hdr->ack = 0;
tcp_hdr->psh = 0;
tcp_hdr->rst = 0;
tcp_hdr->syn = 1;
tcp_hdr->fin = 0;
tcp_hdr->window = htons(1024);
tcp_hdr->check = 0;
tcp_hdr->urg_ptr = 0;
// 发送数据包
data_len = send(sock_fd, buffer, sizeof(struct ethhdr) + sizeof(struct iphdr) + sizeof(struct tcphdr), 0);
if (data_len < 0) {
perror("Error sending packet");
return 1;
}
close(sock_fd);
return 0;
}
```
该程序在基于socket(PF_PACKET,SOCK_RAW,htons(ETH_P_ALL))的基础上,添加了发送数据包的功能。程序首先使用recvfrom函数接收数据包,并解析出IP、TCP头的源地址、目的地址、源端口、目的端口等信息进行输出。然后,程序构造了一个TCP SYN数据包,并使用send函数发送出去,实现了发送数据包的功能。
阅读全文
相关推荐
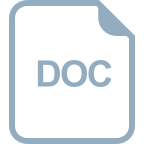
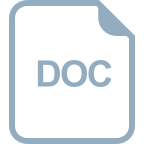
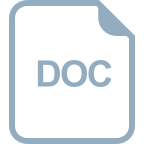
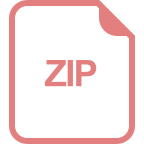
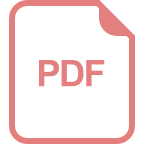
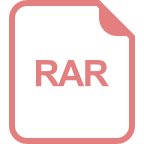
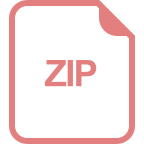
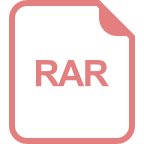
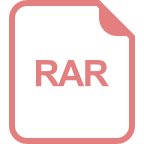
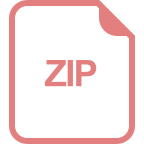
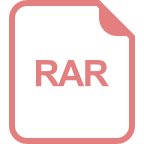
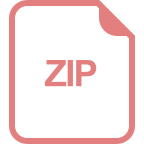
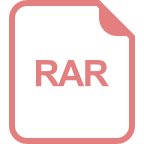
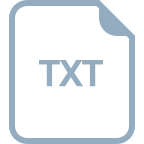
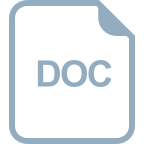
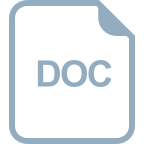