图书管理系统C语言全部代码示例
时间: 2024-10-07 17:04:27 浏览: 31
在C语言中编写一个简单的图书管理系统代码示例可能会包含基本的数据结构如链表来存储书籍信息,以及一些函数用于添加、删除、查找书籍等操作。由于文本格式限制,这里只能提供一个简化的框架代码,你可以根据需求进一步完善。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义图书结构体
typedef struct Book {
char title[100];
char author[100];
int year;
} Book;
// 链表节点
typedef struct Node {
Book book;
struct Node* next;
} Node;
// 初始化链表头指针
Node* list_head = NULL;
// 添加新书
void add_book(char* title, char* author, int year) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("内存分配失败\n");
return;
}
strcpy(newNode->book.title, title);
strcpy(newNode->book.author, author);
newNode->book.year = year;
newNode->next = list_head;
list_head = newNode;
}
// 删除书籍
void delete_book(char* title) {
Node* current = list_head;
Node* previous = NULL;
while (current != NULL && strcmp(current->book.title, title) != 0) {
previous = current;
current = current->next;
}
if (current == NULL) {
printf("未找到该书籍\n");
return;
}
if (previous == NULL) {
list_head = current->next;
} else {
previous->next = current->next;
}
free(current);
}
// 查找书籍
void search_book(char* title) {
Node* current = list_head;
while (current != NULL) {
if (strcmp(current->book.title, title) == 0) {
printf("书名: %s, 作者: %s, 年份: %d\n", current->book.title, current->book.author, current->book.year);
break;
}
current = current->next;
}
}
int main() {
// 示例操作
add_book("红楼梦", "曹雪芹", 1791);
search_book("红楼梦");
delete_book("红楼梦");
return 0;
}
```
阅读全文
相关推荐
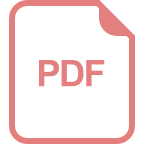
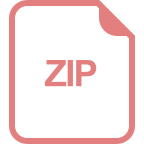
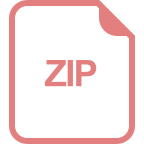
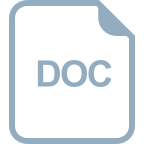
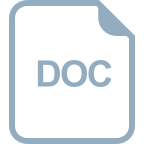
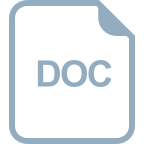






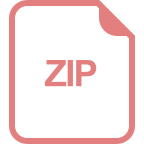
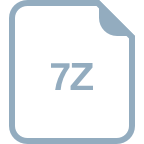
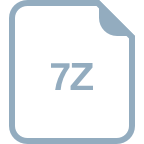
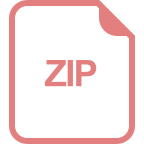
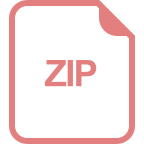