c语言中如何判断一个字符串是不是回文串
时间: 2024-10-01 08:09:16 浏览: 15
在C语言中,判断一个字符串是否是回文串可以通过两种常见的方法实现:
1. **双指针法**:这是一种常用的迭代方法,创建两个指针,一个指向字符串的开始(i),另一个指向结束(j)。然后逐个比较对应的字符,如果它们相等则继续向中间移动,如果不相等则说明不是回文串。当两个指针相遇或者交叉时,都没有发现不相等的字符,则该字符串是回文串。
```c
#include <stdio.h>
#include <string.h>
int isPalindrome(char str[]) {
int i = 0, j = strlen(str) - 1;
while (i < j) {
if (str[i] != str[j])
return 0; // 回文串不成立
i++;
j--;
}
return 1; // 字符串是回文串
}
int main() {
char test[] = "madam";
if (isPalindrome(test))
printf("%s 是回文串\n", test);
else
printf("%s 不是回文串\n", test);
return 0;
}
```
2. **递归法**:另一种方法是递归地比较字符串的第一个字符和最后一个字符,然后递归检查剩下的部分。如果所有对应位置的字符都相等,那么整个字符串就是回文串。
```c
#include <stdbool.h>
#include <string.h>
bool isPalindromeRec(char str[], int start, int end) {
if (start >= end)
return true;
if (str[start] != str[end])
return false;
return isPalindromeRec(str, start + 1, end - 1);
}
// 调用示例
bool isPalindromeCharByChar(const char* str) {
return isPalindromeRec(str, 0, strlen(str) - 1);
}
int main() {
if (isPalindromeCharByChar("madam"))
printf("madam 是回文串\n");
else
printf("madam 不是回文串\n");
return 0;
}
```
相关推荐
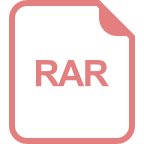
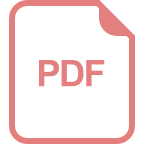














