判断字符串是否为C语言的保留字
时间: 2023-11-15 19:58:42 浏览: 131
为了判断字符串是否为C语言的保留字,我们可以使用以下步骤:
1. 定义一个字符串数组,将C语言的保留字存储在其中。
2. 定义一个函数,该函数接受一个字符串作为参数,并返回一个布尔值。
3. 在函数中,使用循环遍历保留字数组,将每个保留字与传入的字符串进行比较。
4. 如果找到一个匹配的保留字,则返回true,否则返回false。
下面是一个示例代码,可以用来判断字符串是否为C语言的保留字:
```
#include <stdio.h>
#include <string.h>
char *keywords[] = {"auto", "break", "case", "char", "const", "continue", "default", "do", "double", "else", "enum", "extern", "float", "for", "goto", "if", "int", "long", "register", "return", "short", "signed", "sizeof", "static", "struct", "switch", "typedef", "union", "unsigned", "void", "volatile", "while"};
bool isKeyword(char *str) {
int i;
for (i = 0; i < 32; i++) {
if (strcmp(str, keywords[i]) == 0) {
return true;
}
}
return false;
}
```
使用该函数,我们可以轻松地判断一个字符串是否为C语言的保留字。例如:
```
char str1[] = "int";
char str2[] = "hello";
if (isKeyword(str1)) {
printf("%s is a keyword.\n", str1);
} else {
printf("%s is not a keyword.\n", str1);
}
if (isKeyword(str2)) {
printf("%s is a keyword.\n", str2);
} else {
printf("%s is not a keyword.\n", str2);
}
```
输出结果为:
```
int is a keyword.
hello is not a keyword.
```
相关推荐
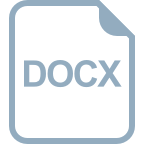
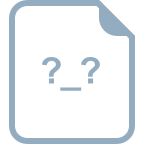
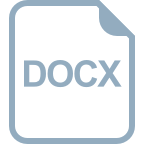














