c语言2) 实现进程管理: a) 假设每个作业只创建一个进程,进程控制块pcb包含进程标
时间: 2023-12-26 20:02:26 浏览: 53
要实现进程管理,首先需要一个进程控制块(PCB)来管理进程相关的信息,包括进程标识、状态、优先级、指令指针等。每个作业创建一个进程时,就会分配一个独特的进程标识,用来识别和管理该进程。
进程的创建可以通过调用fork()函数来实现,通过复制父进程的 PCB 来创建子进程,并分配一个新的进程标识。而进程的销毁则可以通过调用exit()函数来实现,将该进程的 PCB 从进程表中移除,并释放相关资源。
在进程管理中,还需要考虑进程的调度和协作。调度可以通过设置进程的优先级来实现,可以通过调用nice()函数来动态地改变进程的优先级。而进程的协作可以通过使用信号来实现,可以向指定进程发送信号,并由接收进程来响应信号进行相应的处理。
除此之外,通过使用进程间通信机制,例如管道、共享内存、消息队列等,来实现不同进程之间的数据交换和共享。
总之,通过合理地设计和管理进程的 PCB,以及使用各种进程管理的函数和机制,可以很好地实现进程的创建、销毁、调度和协作,从而实现进程管理的功能。
相关问题
c语言实现完成单处理器系统中的进程调度,要求实现时间片轮转、优先数、最短进程优 先和最短剩余时间优先四种调度算法。实验具体包括:首先确定进程控制块的内容,进程控 制块的组成方式;然后完成进程创建原语和进程调度原语;最后编写主函数对所作工作进行 测试。 模拟程序只对你所设置的“虚拟 PCB”进行相应的调度模拟操作,即每发生“调度” 时,显示出当前运行进程的“进程标识符”、“优先数”、“剩余运行时间”等,而不需要对系 统中真正的 PCB 等数据进行修改。要求能够动态地随机生成新进程添加到就绪队列中。主要考虑三个问题:如何组织进程、如何创建进程和如何实现处理器调度。首先要设定进程控制块的内容。进程控制块 PCB 记录各个进程执 行时的情况。每个进程都要有一个唯一的标识符,用来标识进程的存在和区别于其他进程。可以用符号或编号实现,它必须是操作系统分配的。记录进程的基本情况,例如进程的状态、等待原因、进程程序存放位置、进程数据存放位置等等。实验中,因为进程没有数据和程序,仅使用模拟的进程控制块,所以这部分 内容仅包含进程状态。进程状态可假设只有就绪、运行、终止三种。现场信息记录各个寄存器的内容。管理信息记录进程管理和调度的信息。例如进程优先数、进程队列指针等
。以下是一个简单的实现,包括进程控制块的定义、进程创建和调度原语的实现以及四种调度算法的实现。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_PROCESS_NUM 10 // 最大进程数
#define TIME_SLICE 3 // 时间片长度
// 进程状态
enum ProcessState {
READY,
RUNNING,
TERMINATED
};
// 进程控制块
struct PCB {
int pid; // 进程标识符
enum ProcessState state; // 进程状态
int priority; // 进程优先级
int arrival_time; // 进程到达时间
int remaining_time; // 进程剩余执行时间
};
// 就绪队列
struct PCB *ready_queue[MAX_PROCESS_NUM];
int ready_queue_size = 0;
// 当前运行进程
struct PCB *running_process = NULL;
// 进程标识符计数器
int pid_counter = 0;
// 随机生成进程
void generate_process() {
// 进程数量已达到最大值,不再生成新进程
if (ready_queue_size == MAX_PROCESS_NUM) {
return;
}
// 生成新的进程控制块
struct PCB *process = (struct PCB *) malloc(sizeof(struct PCB));
process->pid = pid_counter++;
process->state = READY;
process->priority = rand() % 5; // 随机生成优先级
process->arrival_time = rand() % 10; // 随机生成到达时间
process->remaining_time = rand() % 10 + 1; // 随机生成剩余执行时间
// 将新进程加入就绪队列
ready_queue[ready_queue_size++] = process;
}
// 时间片轮转调度算法
void schedule_round_robin() {
// 当前没有运行进程,从就绪队列中选取一个进程开始执行
if (running_process == NULL) {
if (ready_queue_size > 0) {
running_process = ready_queue[0];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = 0; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
// 当前有运行进程,时间片到期,将其放回就绪队列尾部
else {
running_process->remaining_time -= TIME_SLICE;
if (running_process->remaining_time <= 0) {
running_process->state = TERMINATED;
printf("Terminating process %d\n", running_process->pid);
free(running_process);
running_process = NULL;
}
else {
running_process->state = READY;
printf("Putting process %d back to ready queue (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue[ready_queue_size++] = running_process;
running_process = NULL;
}
}
}
// 优先数调度算法
void schedule_priority() {
// 从就绪队列中选取优先级最高的进程开始执行
int highest_priority = -1;
int highest_priority_index = -1;
for (int i = 0; i < ready_queue_size; i++) {
if (ready_queue[i]->priority > highest_priority) {
highest_priority = ready_queue[i]->priority;
highest_priority_index = i;
}
}
if (highest_priority_index != -1) {
running_process = ready_queue[highest_priority_index];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = highest_priority_index; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
// 最短进程优先调度算法
void schedule_shortest_job_first() {
// 从就绪队列中选取需要执行时间最短的进程开始执行
int shortest_time = 100000;
int shortest_time_index = -1;
for (int i = 0; i < ready_queue_size; i++) {
if (ready_queue[i]->remaining_time < shortest_time) {
shortest_time = ready_queue[i]->remaining_time;
shortest_time_index = i;
}
}
if (shortest_time_index != -1) {
running_process = ready_queue[shortest_time_index];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = shortest_time_index; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
// 最短剩余时间优先调度算法
void schedule_shortest_remaining_time_first() {
// 从就绪队列中选取需要执行时间最短的进程开始执行
int shortest_time = 100000;
int shortest_time_index = -1;
for (int i = 0; i < ready_queue_size; i++) {
if (ready_queue[i]->remaining_time < shortest_time) {
shortest_time = ready_queue[i]->remaining_time;
shortest_time_index = i;
}
}
if (shortest_time_index != -1) {
// 如果当前没有运行进程,或者运行进程的剩余执行时间比被选中的进程剩余执行时间多,
// 则将被选中的进程作为当前运行进程,否则将其放回就绪队列尾部
if (running_process == NULL || running_process->remaining_time > shortest_time) {
if (running_process != NULL) {
running_process->state = READY;
printf("Putting process %d back to ready queue (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue[ready_queue_size++] = running_process;
}
running_process = ready_queue[shortest_time_index];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = shortest_time_index; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
}
// 主函数
int main() {
srand(time(NULL));
// 生成初始进程
for (int i = 0; i < MAX_PROCESS_NUM / 2; i++) {
generate_process();
}
// 模拟进程调度
for (int i = 0; i < 20; i++) {
printf("Time %d:\n", i);
// 随机生成新进程
if (rand() % 2 == 0) {
generate_process();
}
// 执行调度算法
//schedule_round_robin();
//schedule_priority();
//schedule_shortest_job_first();
schedule_shortest_remaining_time_first();
}
return 0;
}
```
注意,这个实现仅是一个简单的示例,实际的操作系统中还需要考虑更多的因素,例如进程间的同步与通信、内存管理、文件系统等等。
写出时间片轮转调度算法的C语言代码,具体要求如下: 1.数据结构设计 PCB结构: name 进程名 round 进程轮转时间片 cputime 进程占用的CPU时间 needtime 进程到完成还要的时间 state 进程状态(假设状态为Ready、Run、Finish) next
这是一个简单的时间片轮转调度算法的C语言代码实现,代码中使用了链表来存储进程控制块(PCB):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LENGTH 20
#define TIME_SLICE 2
// 进程状态定义
typedef enum {Ready, Run, Finish} State;
// 进程控制块(PCB)结构体
typedef struct pcb {
char name[MAX_NAME_LENGTH];
int round;
int cputime;
int needtime;
State state;
struct pcb *next;
} PCB;
// 初始化进程控制块
void init_pcb(PCB *pcb, char *name, int round, int needtime) {
strcpy(pcb->name, name);
pcb->round = round;
pcb->cputime = 0;
pcb->needtime = needtime;
pcb->state = Ready;
pcb->next = NULL;
}
// 插入进程控制块到链表尾部
void insert_pcb(PCB **head, PCB *pcb) {
if (*head == NULL) {
*head = pcb;
} else {
PCB *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = pcb;
}
}
// 从链表中删除进程控制块
void delete_pcb(PCB **head, PCB *pcb) {
if (*head == NULL) {
return;
}
if (*head == pcb) {
*head = pcb->next;
} else {
PCB *p = *head;
while (p->next != NULL && p->next != pcb) {
p = p->next;
}
if (p->next == pcb) {
p->next = pcb->next;
}
}
}
// 时间片轮转调度算法
void round_robin_scheduling(PCB **head) {
if (*head == NULL) {
return;
}
PCB *p = *head;
while (p != NULL) {
if (p->state == Run) {
p->cputime += TIME_SLICE;
p->needtime -= TIME_SLICE;
if (p->needtime <= 0) {
p->state = Finish;
printf("Process %s finished.\n", p->name);
}
else if (p->cputime >= p->round) {
p->state = Ready;
p->cputime = 0;
printf("Process %s time slice ended.\n", p->name);
}
break;
}
p = p->next;
}
if (p == NULL) {
p = *head;
} else {
p = p->next;
}
while (p != *head) {
if (p->state == Ready) {
p->state = Run;
printf("Process %s is running.\n", p->name);
break;
}
p = p->next;
}
if (p == *head && p->state != Run) {
printf("No process to run.\n");
}
}
// 打印进程状态
void print_state(PCB *pcb) {
switch (pcb->state) {
case Ready:
printf("Ready");
break;
case Run:
printf("Run");
break;
case Finish:
printf("Finish");
break;
}
}
// 打印所有进程状态
void print_all_state(PCB *head) {
PCB *p = head;
while (p != NULL) {
printf("Process %s, round %d, cputime %d, needtime %d, state ", p->name, p->round, p->cputime, p->needtime);
print_state(p);
printf("\n");
p = p->next;
}
}
int main() {
PCB *head = NULL;
PCB *p1 = (PCB *)malloc(sizeof(PCB));
init_pcb(p1, "Process1", 4, 10);
insert_pcb(&head, p1);
PCB *p2 = (PCB *)malloc(sizeof(PCB));
init_pcb(p2, "Process2", 2, 8);
insert_pcb(&head, p2);
PCB *p3 = (PCB *)malloc(sizeof(PCB));
init_pcb(p3, "Process3", 3, 6);
insert_pcb(&head, p3);
print_all_state(head);
printf("\n");
while(head != NULL) {
round_robin_scheduling(&head);
print_all_state(head);
printf("\n");
}
return 0;
}
```
这段代码实现了一个包含三个进程的时间片轮转调度算法。在运行时,程序会输出每个进程的状态,包括进程名、轮转时间片、已占用的CPU时间、剩余需要的CPU时间和状态(Ready、Run、Finish)。每次调度后,程序会输出当前正在运行的进程名和所有进程的状态。如果没有进程可以运行了,则输出“No process to run.”。
相关推荐
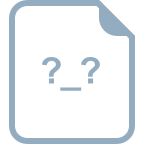
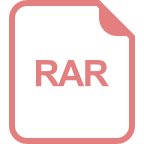
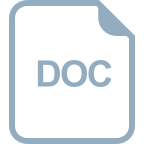



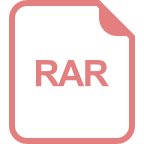
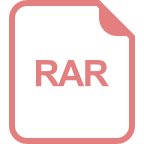
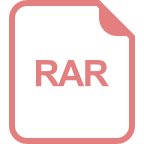
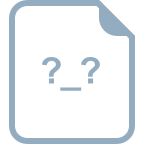
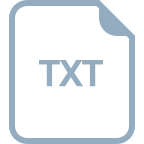
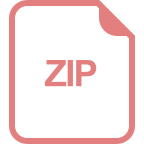
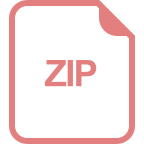