在vue中在实体将时间格式为yyyy-MM
时间: 2024-09-25 16:06:44 浏览: 31
在 Vue.js 中,如果你有一个实体对象需要将日期时间格式化为 "yyyy-MM" 格式,你可以利用 JavaScript 的内置 `Date` 对象配合字符串模板或库如 moment.js 或者简单的正则表达式来进行转换。这里是一个简单示例:
```javascript
// 假设你有一个名为item的实体对象,其中包含一个date属性
let item = {
date: new Date() // 这里假设date属性是当前日期
}
// 使用JavaScript原生的方法
let formattedDate = item.date.getFullYear() + '-' + (item.date.getMonth() + 1).toString().padStart(2, '0');
// 如果你使用了moment.js库
import moment from 'moment';
let formattedDateMoment = moment(item.date).format('YYYY-MM');
// 如果你想直接操作字符串
let dateString = item.date.toString();
formattedDate = dateString.slice(0, 4) + '-' + dateString.slice(5, 7);
相关问题
用springboot,vue,mybatis和elementUI实现上传PDF文件到数据库,需要上传到数据库的内容有文件名,文件上传时间,文件路径,上传者的编号。请给我实体类代码,控制器代码,前端代码,mybatis代码和数据库建表语句
### 回答1:
很高兴为您提供所需的代码和建表语句。
首先,这是实体类代码:
```
public class PdfFile {
private Long id;
private String fileName;
private String filePath;
private Date uploadTime;
private Long uploaderId;
// getters and setters
}
```
接下来是控制器代码:
```
@RestController
@RequestMapping("/pdf")
public class PdfController {
@Autowired
private PdfService pdfService;
@PostMapping("/upload")
public ResponseEntity<?> uploadPdf(@RequestParam("file") MultipartFile file, @RequestParam("uploaderId") Long uploaderId) {
try {
PdfFile pdfFile = pdfService.savePdf(file, uploaderId);
return ResponseEntity.ok(pdfFile);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
```
然后是前端代码:
```
<template>
<div>
<input type="file" @change="onFileChange">
<button @click="uploadPdf">上传</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null,
uploaderId: null
};
},
methods: {
onFileChange(event) {
this.file = event.target.files[0];
},
uploadPdf() {
const formData = new FormData();
formData.append('file', this.file);
formData.append('uploaderId', this.uploaderId);
axios.post('/pdf/upload', formData)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
}
}
};
</script>
```
接下来是 MyBatis 的代码:
```
@Mapper
public interface PdfMapper {
@Insert("INSERT INTO pdf_file (file_name, file_path, upload_time, uploader_id) VALUES (#{fileName}, #{filePath}, #{uploadTime}, #{uploaderId})")
@Options(useGeneratedKeys = true, keyProperty = "id")
void insertPdf(PdfFile pdfFile);
}
```
最后是建表语句:
```
CREATE TABLE pdf_file (
id BIGINT PRIMARY KEY AUTO_INCREMENT,
file_name VARCHAR(255) NOT NULL,
file_path VARCHAR(255) NOT NULL,
upload_time DATETIME NOT NULL,
uploader_id BIGINT NOT NULL
);
```
希望这些代码能够帮助您实现上传 PDF 文件到数据库的功能。
### 回答2:
首先是实体类代码:
```java
// 文件实体类
public class FileEntity {
private String fileName;
private String uploadTime;
private String filePath;
private int uploaderId;
// 省略getter和setter方法
}
```
接下来是控制器代码(使用Spring Boot):
```java
@RestController
public class FileController {
@Autowired
private FileService fileService;
@PostMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file, @RequestParam("uploaderId") int uploaderId) {
// 生成文件保存路径
// ...
// 保存文件到指定路径
// ...
// 构建文件实体类
FileEntity fileEntity = new FileEntity();
fileEntity.setFileName(file.getOriginalFilename());
fileEntity.setUploadTime(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(new Date()));
fileEntity.setFilePath(filePath);
fileEntity.setUploaderId(uploaderId);
// 调用Service层保存文件实体类到数据库
fileService.saveFile(fileEntity);
return "文件上传成功";
}
}
```
再来是前端代码(使用Vue和ElementUI):
```html
<template>
<div>
<input type="file" ref="file" @change="handleFileChange">
<el-button type="primary" @click="uploadFile">上传文件</el-button>
</div>
</template>
<script>
export default {
data() {
return {
file: null
};
},
methods: {
handleFileChange(e) {
this.file = e.target.files[0];
},
uploadFile() {
let formData = new FormData();
formData.append("file", this.file);
formData.append("uploaderId", 123); // 上传者的编号
this.$http.post("/uploadFile", formData).then(response => {
console.log(response.data);
// 文件上传成功的操作
});
}
}
};
</script>
```
接下来是MyBatis的代码(使用注解方式):
```java
@Mapper
public interface FileMapper {
@Insert("INSERT INTO file (file_name, upload_time, file_path, uploader_id) VALUES (#{fileName}, #{uploadTime}, #{filePath}, #{uploaderId})")
void saveFile(FileEntity fileEntity);
}
```
最后是数据库的建表语句:
```sql
CREATE TABLE file (
id INT AUTO_INCREMENT PRIMARY KEY,
file_name VARCHAR(255) NOT NULL,
upload_time DATETIME NOT NULL,
file_path VARCHAR(255) NOT NULL,
uploader_id INT NOT NULL
);
```
### 回答3:
实体类代码:
```java
// 文件实体类
public class FileEntity {
private Integer id;
private String fileName;
private Date uploadTime;
private String filePath;
private Integer uploaderId;
// 省略getter和setter方法
}
```
控制器代码:
```java
@RestController
public class FileController {
@Autowired
private FileService fileService;
@PostMapping("/uploadFile")
public String uploadFile(MultipartFile file, Integer uploaderId) {
try {
String fileName = file.getOriginalFilename();
String filePath = "指定上传文件的保存路径";
FileEntity fileEntity = new FileEntity();
fileEntity.setFileName(fileName);
fileEntity.setUploadTime(new Date());
fileEntity.setFilePath(filePath);
fileEntity.setUploaderId(uploaderId);
fileService.uploadFile(fileEntity);
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
}
}
```
前端代码:
```vue
<template>
<div>
<input type="file" @change="handleFileChange" />
<button @click="uploadFile">上传</button>
</div>
</template>
<script>
export default {
data() {
return {
file: null
};
},
methods: {
handleFileChange(e) {
this.file = e.target.files[0];
},
uploadFile() {
const formData = new FormData();
formData.append("file", this.file);
formData.append("uploaderId", 1); // 上传者的编号
// 使用axios或其他方式发送POST请求,将formData作为参数传递给后端接口
}
}
}
</script>
```
MyBatis代码:
```xml
<!-- Mapper接口 -->
public interface FileMapper {
void insertFile(FileEntity fileEntity);
}
<!-- Mapper.xml文件 -->
<insert id="insertFile" parameterType="com.example.entity.FileEntity">
INSERT INTO file (file_name, upload_time, file_path, uploader_id)
VALUES (#{fileName}, #{uploadTime}, #{filePath}, #{uploaderId})
</insert>
```
数据库建表语句:
```sql
CREATE TABLE `file` (
`id` INT(11) PRIMARY KEY AUTO_INCREMENT,
`file_name` VARCHAR(255) NOT NULL,
`upload_time` DATETIME NOT NULL,
`file_path` VARCHAR(255) NOT NULL,
`uploader_id` INT(11) NOT NULL
);
```
vue怎么传LocalDateTime
要在Vue中传递LocalDateTime,你可以将其转换为字符串,并在请求或响应中传递。在后台的实体类中,你可以使用@DateTimeFormat注解来指定日期的格式。比如,在后台的实体类的日期字段上添加@DateTimeFormat注解,使用pattern属性指定日期的格式,例如"yyyy-MM-dd HH:mm:ss"。然后,在Vue中,你可以通过将LocalDateTime格式化为字符串来传递。例如,你可以使用moment.js库将LocalDateTime格式化为指定的字符串格式,然后在请求或响应中传递这个字符串。这样就可以在Vue中正确传递LocalDateTime了。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [vue+SpringBoob前端往后端传时间](https://blog.csdn.net/pleaseprintf/article/details/128762459)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
阅读全文
相关推荐













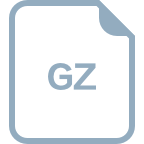

