v4l2 opencv qt
时间: 2023-09-01 16:12:05 浏览: 49
V4L2 (Video for Linux 2) is a kernel API that provides an interface for capturing and manipulating video devices on Linux. OpenCV is a widely used computer vision library that provides various functionalities for image and video processing. Qt is a cross-platform application framework that allows you to develop graphical user interfaces.
If you want to work with V4L2, OpenCV, and Qt together, you can use OpenCV to capture video frames from a V4L2 device and then use Qt to display the captured frames in a graphical user interface. Here are the steps you can follow:
1. Install the necessary libraries:
- Install V4L2 library: `sudo apt-get install libv4l-dev`
- Install OpenCV library: You can either download it from the official website or install it using package manager (e.g., `pip install opencv-python`)
2. Include the required headers in your C++ code:
```cpp
#include <linux/videodev2.h> // V4L2 headers
#include <opencv2/opencv.hpp> // OpenCV headers
#include <QtWidgets/QApplication> // Qt headers
```
3. Use V4L2 to capture video frames:
```cpp
int fd;
fd = open("/dev/video0", O_RDWR); // Open the V4L2 device
// Set up video capture parameters
struct v4l2_format fmt;
// ...
// Request buffers from the V4L2 device
struct v4l2_requestbuffers reqbuf;
// ...
// Queue the buffers for capturing
struct v4l2_buffer buf;
// ...
// Start capturing frames
enum v4l2_buf_type type = V4L2_BUF_TYPE_VIDEO_CAPTURE;
ioctl(fd, VIDIOC_STREAMON, &type);
// Capture frames
for (int i = 0; i < numFrames; ++i) {
// Dequeue a buffer
// ...
// Process the captured frame using OpenCV
cv::Mat frame;
// ...
// Display the frame using Qt
QImage image(frame.data, frame.cols, frame.rows, QImage::Format_RGB888);
// ...
}
// Cleanup and close the V4L2 device
// ...
```
4. Use Qt to display the frames:
```cpp
QApplication app(argc, argv);
QWidget window;
QLabel label(&window);
label.setFixedSize(frame.cols, frame.rows);
label.setPixmap(QPixmap::fromImage(image));
label.show();
return app.exec();
```
Remember to handle error checking, memory management, and other necessary operations according to your application's needs.
相关推荐
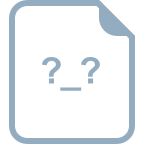














