将字符串中数字提取出来从大到小排列C语言
时间: 2024-11-25 17:18:24 浏览: 4
在C语言中,你可以使用标准库函数和一些自定义函数来提取字符串中的数字,并将它们转换为整数,最后按照从大到小的顺序排序。这里是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <string.h>
// 函数来检查字符是否为数字
int is_digit(char c) {
return (isdigit(c));
}
// 函数用于处理单个数字并添加到数组中
void add_number(char *str, int *numbers, int count) {
int num = 0;
while (is_digit(*str)) {
num = num * 10 + (*str - '0');
str++;
}
if (num > 0) { // 如果不是空的数字
numbers[count++] = num;
}
}
// 主函数来提取并排序数字
void sort_numbers(char *str) {
int numbers[10], count = 0;
char *end = NULL;
while ((end = strchr(str, '\0')) != NULL) {
while (str < end && !is_digit(*str)) {
str++; // 跳过非数字字符
}
if (str < end) {
add_number(str, numbers, count);
str = end; // 移动指针到下一个可能的数字开始位置
}
}
qsort(numbers, count, sizeof(int), compare_ints); // 使用快速排序对数组进行降序排序
}
// 比较函数,用于qsort中的降序排列
int compare_ints(const void *a, const void *b) {
return (*(const int *)b - *(const int *)a);
}
int main() {
char input[] = "abc123def456ghijkl789";
sort_numbers(input);
printf("Sorted numbers from large to small: ");
for (int i = 0; i < 9; i++) {
printf("%d ", numbers[i]);
}
return 0;
}
```
这个程序首先遍历字符串,找到每个连续的数字部分,然后将其添加到数组中。数组会被排序,以便显示从大到小的数字。
阅读全文
相关推荐
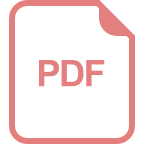
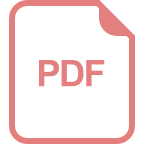
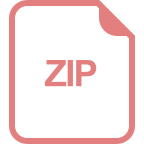
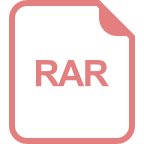
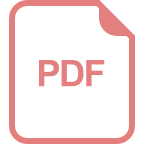
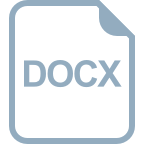
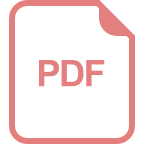
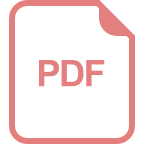
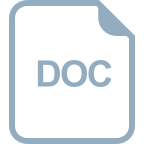
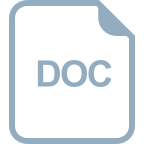
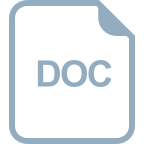
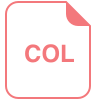
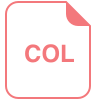
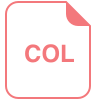



