set如何查询某元素位置c++
时间: 2024-09-23 15:14:33 浏览: 38
在C++中,`set`是一种关联容器,它存储的是唯一的、有序的键值对(key-value pairs),而不是特定类型的元素的位置。`set`内部是通过红黑树数据结构实现的,其主要关注的是查找、插入和删除操作的速度,而不是元素的顺序或者索引。
如果你需要查询某个元素是否存在于`set`中,你可以使用`find`函数,例如:
```cpp
#include <set>
std::set<int> mySet; // 初始化一个整数集合
// 检查元素是否存在
int target = 5;
if (mySet.find(target) != mySet.end()) {
std::cout << "元素 " << target << " 存在于集合中.\n";
} else {
std::cout << "元素 " << target << " 不存在于集合中.\n";
}
```
然而,`set`并不支持直接获取元素的索引或位置,因为它是无序的,并且不保证元素的连续存储。如果你需要按照特定条件访问元素,可能需要将其转换为其他容器如`vector`,并维护额外的数据结构来跟踪元素的顺序。
相关问题
c++set如何插入元素
在 C 语言中,可以使用指针和数组来插入元素。以下是两种常见的方法:
1. 使用数组:可以通过循环将新元素插入到数组中,并移动后续元素的位置。例如,要在数组 a 中插入一个元素 x 到索引 i:
```
int a[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int x = 100;
int i = 3;
int len = 10;
// 在 index i 插入元素 x
len++; // 数组长度增加 1
for (int j = len - 1; j > i; j--) {
a[j] = a[j - 1]; // 移动后续元素
}
a[i] = x; // 插入元素 x
```
2. 使用指针:可以创建一个新节点,并将它插入到链表的适当位置。例如,要在一个单向链表中插入一个值 x 到节点 p 之后:
```
struct Node {
int val;
struct Node* next;
};
struct Node* p = ...; // 需要插入元素节点的前一个节点
int x = 100;
// 创建新节点并初始化
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->val = x;
// 插入新节点
new_node->next = p->next;
p->next = new_node;
```
用set怎么实现优先队列c++
在C++中,标准库并没有直接提供优先队列(Priority Queue),但是你可以通过`std::priority_queue`容器配合自定义的比较函数或适配器来实现一个简单的优先级排序的功能。`std::priority_queue`默认使用最大堆(即最小元素位于根节点),如果你想实现一个最小堆(即最大元素在根节点),可以使用`std::make_heap`、`push_heap`和`pop_heap`等函数手动管理堆。
以下是一个基本的示例,展示如何创建一个基于`int`类型的最小优先队列:
```cpp
#include <queue>
#include <vector>
// 自定义一个比较函数,用于确定元素的优先级
bool compare(const int& a, const int& b) {
return a > b; // 如果需要最大值优先,改为 a < b
}
class CustomPriorityQueue {
private:
std::vector<int> pq;
bool is_heap;
public:
void push(int value) {
pq.push(value);
if (pq.size() > 1) {
// 将最后一个元素调整到堆顶位置
std::push_heap(pq.begin(), pq.end(), compare);
is_heap = true;
}
}
int top() {
if (is_heap) {
return pq.front(); // 返回堆顶元素(当前最高优先级)
} else {
throw std::runtime_error("Priority queue is empty or not maintained as heap.");
}
}
void pop() {
if (is_heap) {
pq.pop();
if (!pq.empty()) {
// 移除堆顶元素后,重新调整堆
std::pop_heap(pq.begin(), pq.end(), compare);
pq.pop();
is_heap = pq.size() > 1;
if (is_heap) {
std::push_heap(pq.begin(), pq.end(), compare);
}
} else {
is_heap = false;
}
} else {
throw stdruntime_error("Priority queue is empty or not maintained as heap.");
}
}
};
阅读全文
相关推荐
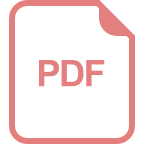
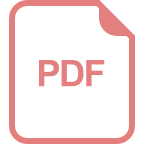
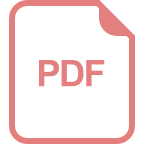
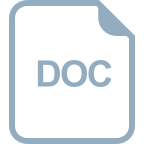
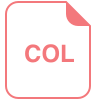











