如何用C++语言实现AES加密算法的具体步骤和代码示例?
时间: 2024-10-27 08:13:42 浏览: 128
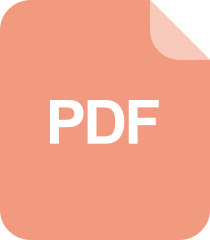
C++中四种加密算法之AES源代码
AES(Advanced Encryption Standard,高级加密标准)是一种对称密钥加密算法,用于保护数据隐私。在C++中实现AES加密通常需要依赖于第三方库,如Crypto++或Poco等,因为直接手写AES算法较为复杂。以下是使用Crypto++库的一个简单示例:
首先,你需要包含必要的头文件,并确保已经安装了Crypto++库:
```cpp
#include <cryptopp/aes.h>
#include <cryptopp/modes.h>
#include <cryptopp/filters.h>
```
然后创建一个函数,用于初始化AES上下文并加密数据:
```cpp
using namespace CryptoPP;
void aes_encrypt(const std::string& key, const std::string& plaintext, std::string& ciphertext) {
byte keyByte[32]; // AES uses 128-bit keys (32 bytes)
StringSource(plaintext, true,
new PKCS7Padding(),
new ByteSource(keyByte, sizeof(keyByte)),
new CBC_Mode_ExternalCipher(AES::Encryption(), keyByte),
new StreamTransformationFilter(new HexEncoder(), ciphertext));
}
```
这里的关键点解释一下:
1. `PKCS7Padding`添加PKCS#7填充,确保输入数据长度为AES块大小的倍数。
2. `CBC_Mode_ExternalCipher`使用密码模式(Cipher Block Chaining),提供了一个外部加密操作的封装。
3. `HexEncoder`将最终的二进制密文转换成十六进制字符串。
为了使用这个函数,你需要传递一个16字节的密钥和明文,它会返回一个十六进制的密文字符串:
```cpp
std::string myKey = "your_secret_key"; // 替换为实际的16字节密钥
std::string plaintext = "Hello, world!";
std::string ciphertext;
aes_encrypt(myKey, plaintext, ciphertext);
```
请注意,这只是一个基本示例,实际使用时还需要处理错误、内存管理和安全等问题。如果你需要更详细的指导或使用其他库,请查阅对应文档。
阅读全文
相关推荐
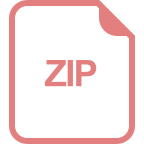
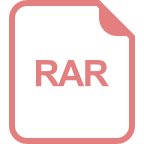
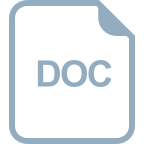
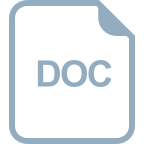
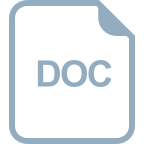
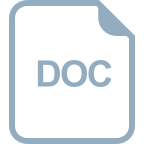
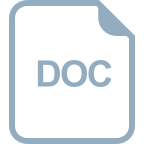

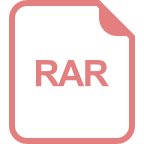
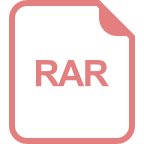
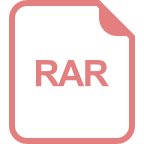
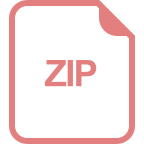
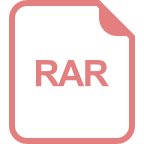
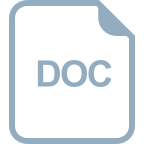
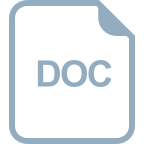
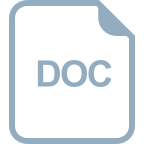

