rbf神经网络二分类小批量训练
时间: 2023-09-04 10:16:42 浏览: 94
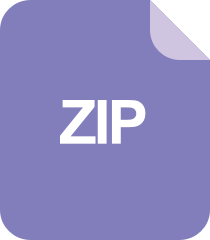
RBF神经网络的训练
RBF神经网络的小批量训练是一种常用的训练方法,它可以加快训练速度并提高收敛性能。下面是一个使用小批量训练的RBF神经网络二分类示例:
首先,导入所需的库和模块:
```python
import numpy as np
from sklearn.preprocessing import StandardScaler
from sklearn.datasets import make_classification
```
然后,生成一个示例数据集进行训练:
```python
# 生成示例数据集
X, y = make_classification(n_samples=1000, n_features=10, n_classes=2, random_state=42)
# 数据预处理
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
```
接下来,定义RBF神经网络的相关函数和参数:
```python
def gaussian_rbf(x, c, s):
return np.exp(-np.linalg.norm(x-c)**2 / (2 * s**2))
def initialize_centers(X, k):
random_indices = np.random.choice(range(X.shape[0]), size=k)
centers = X[random_indices]
return centers
def compute_activations(X, centers, sigma):
A = np.zeros((X.shape[0], centers.shape[0]))
for i, x in enumerate(X):
for j, c in enumerate(centers):
A[i, j] = gaussian_rbf(x, c, sigma)
return A
```
然后,定义小批量训练的函数:
```python
def minibatch_train(X, y, k, sigma, lr, epochs, batch_size):
centers = initialize_centers(X, k)
for epoch in range(epochs):
indices = np.random.permutation(X.shape[0])
X = X[indices]
y = y[indices]
for i in range(0, X.shape[0], batch_size):
X_batch = X[i:i+batch_size]
y_batch = y[i:i+batch_size]
A = compute_activations(X_batch, centers, sigma)
W = np.linalg.inv(A.T @ A) @ A.T @ y_batch
y_pred = A @ W
error = y_pred - y_batch
delta_W = - lr * (A.T @ error) / batch_size
centers -= lr * (W.reshape(-1, 1) * (X_batch - centers)) / (sigma**2 * batch_size)
return centers
```
最后,调用小批量训练函数进行训练并进行预测:
```python
k = 10 # 隐藏层神经元数量
sigma = 1.0 # 高斯函数的标准差
lr = 0.01 # 学习率
epochs = 100 # 迭代次数
batch_size = 32 # 批量大小
# 小批量训练
centers = minibatch_train(X_scaled, y, k, sigma, lr, epochs, batch_size)
# 计算隐藏层的输出
A_test = compute_activations(X_scaled, centers, sigma)
# 预测测试集
y_pred = np.sign(A_test)
# 计算准确率
accuracy = np.mean(y_pred == y)
```
这是一个简单的使用小批量训练的RBF神经网络二分类示例。你可以根据需要调整参数和增加其他功能来进一步改进模型。
阅读全文
相关推荐
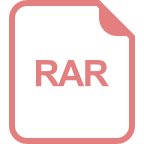
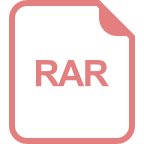
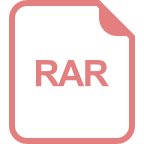
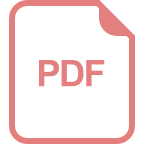
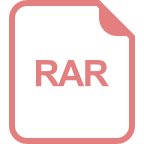
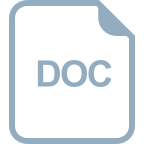
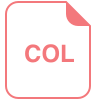
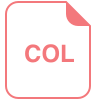
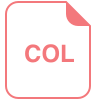
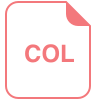
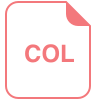
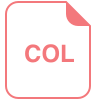
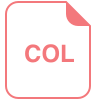
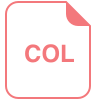
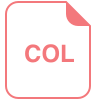
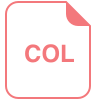
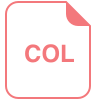