LSTMpython
时间: 2023-10-28 10:59:38 浏览: 110
LSTM是一种深度学习模型,它在处理序列数据时比传统的循环神经网络(RNN)更具优势。在Python中,我们可以使用不同的库来实现LSTM模型,如TensorFlow、Keras和PyTorch。通过这些库,我们可以创建LSTM网络并训练模型以适应特定的任务,例如文本分类或情感分析。
在Python中使用LSTM模型通常涉及以下步骤:
1. 导入所需的库,例如TensorFlow或Keras。
2. 准备数据集,包括数据预处理、分割训练集和测试集等。
3. 构建LSTM模型,可以使用库中提供的LSTM层或自定义LSTM结构。
4. 编译模型,指定损失函数、优化器和评估指标。
5. 训练模型,使用训练集进行模型的训练,并根据验证集的表现来调整模型参数。
6. 评估模型,使用测试集对模型进行评估,计算模型的准确率、精度、召回率等指标。
7. 使用模型进行预测,将新的数据输入模型中,获取预测结果。
以上是使用LSTM模型进行文本分类的一般步骤,具体的实现可以根据实际情况来调整。你可以参考中提供的相关参考资料了解更多关于LSTM在Python中的实现方法。
相关问题
Lstm Python
LSTM (Long Short-Term Memory) is a type of recurrent neural network that is widely used for analyzing sequential data, such as time series or text data. In Python, you can implement LSTM using various libraries such as Keras, PyTorch, or TensorFlow. Here is an example of how to implement LSTM using Keras:
```
from keras.models import Sequential
from keras.layers import LSTM, Dense
model = Sequential()
model.add(LSTM(64, input_shape=(timesteps, input_dim)))
model.add(Dense(num_classes, activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10, batch_size=32)
```
In this example, we create a sequential model that consists of an LSTM layer with 64 units and a dense layer with softmax activation. We compile the model with categorical cross-entropy loss and the Adam optimizer, and then fit the model using the training data.
Note that `timesteps` and `input_dim` in the input shape depend on the shape of your data. For example, if you have a time series of length 100 with 3 features at each time step, then `timesteps` would be 100 and `input_dim` would be 3.
lstm python
### 回答1:
LSTM(Long-Short Term Memory)是一种常用的循环神经网络(RNN)架构,用于处理序列数据。在Python中,可以使用多个深度学习框架如TensorFlow、Keras、PyTorch等来实现LSTM模型。
以下是一个简单的Python代码示例,演示如何使用Keras实现LSTM模型:
```python
from keras.models import Sequential
from keras.layers import LSTM, Dense
# 创建LSTM模型
model = Sequential()
model.add(LSTM(64, input_shape=(X_train.shape[1], X_train.shape[2])))
model.add(Dense(1))
# 编译模型
model.compile(loss='mae', optimizer='adam')
# 训练模型
model.fit(X_train, y_train, epochs=100, batch_size=16, validation_data=(X_test, y_test))
```
在上面的代码示例中,我们首先从Keras中导入Sequential、LSTM和Dense类。接下来,我们使用Sequential类创建一个神经网络模型,并向其中添加一个LSTM层和一个全连接层。然后,我们使用compile()方法配置模型的损失函数和优化器,并使用fit()方法训练模型。
当然,这只是LSTM模型的一个简单示例。如果你想更深入地了解LSTM模型的实现和应用,建议你阅读更多的资料和文献。
### 回答2:
LSTM(长短期记忆网络)是一种常用于处理序列数据的深度学习模型。它是一种带有门控机制的循环神经网络(RNN)的变体,通过引入门控单元来解决长序列训练中的梯度消失问题。在Python中,我们可以使用多种深度学习库实现LSTM模型,如TensorFlow、PyTorch、Keras等。
首先,我们需要导入相关的库。在使用TensorFlow时,可以使用以下命令导入LSTM模型相关的库:
```python
import tensorflow as tf
from tensorflow.keras.layers import LSTM, Dense
from tensorflow.keras.models import Sequential
```
接下来,我们可以定义一个LSTM模型。一个基本的LSTM模型可以包含一个或多个LSTM层和输出层。以下是一个简单的LSTM模型的示例:
```python
model = Sequential()
model.add(LSTM(128, input_shape=(timesteps, input_dim)))
model.add(Dense(num_classes, activation='softmax'))
```
在这个示例中,我们首先创建了一个Sequential模型。然后,使用add方法依次添加一个LSTM层和一个全连接输出层。LSTM层的参数包括单元数(128)、输入形状(timesteps、input_dim)等。全连接输出层则定义了输出的类别数和激活函数。
模型的训练可以通过以下步骤完成:
```python
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
model.fit(X_train, y_train, batch_size=batch_size, epochs=epochs, validation_data=(X_val, y_val))
```
在这个例子中,我们使用了交叉熵作为损失函数、Adam优化器,并使用训练数据(X_train和y_train)进行训练。设置了批量大小(batch_size)和迭代次数(epochs)。还可以通过添加验证数据(validation_data)进行模型的验证。
最后,我们可以使用模型对新数据进行预测:
```python
y_pred = model.predict(X_test)
```
以上就是使用Python实现LSTM模型的简单步骤。当然,LSTM模型还有许多高级用法和更多的参数配置,可以根据具体的需求进行调整和优化。
阅读全文
相关推荐
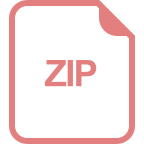

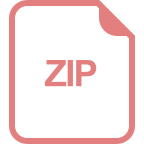
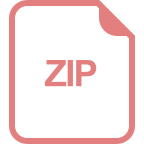
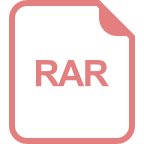








