C#中ZipFile.Open
时间: 2023-11-26 17:45:49 浏览: 40
C#中的ZipFile.Open方法是用于打开一个ZIP文件并返回一个ZipArchive对象,以便对ZIP文件进行读取或写入操作。该方法有多个重载,可以根据需要选择不同的参数。例如,可以使用ZipFile.Open方法创建一个新的ZIP文件,也可以打开现有的ZIP文件进行读取或修改。在使用ZipFile.Open方法之前,需要确保已经添加了System.IO.Compression和System.IO.Compression.FileSystem的引用。
相关问题
C# 判断压缩文件是否损坏
在 C# 中,我们可以使用 System.IO.Compression 命名空间提供的 ZipArchive 类来判断压缩文件是否损坏。具体操作步骤如下:
1. 引入命名空间:using System.IO.Compression;
2. 创建 ZipArchive 对象:ZipArchive archive = ZipFile.OpenRead("yourZipFile.zip");
3. 遍历压缩包中的所有条目,检查是否有损坏的文件:foreach (ZipArchiveEntry entry in archive.Entries) { if (!entry.FullName.EndsWith("/")) { entry.Open().Close(); } }
如果遍历到某个条目时出现异常,则说明该条目所对应的文件已经损坏,需要进行相应的处理。例如,可以记录日志、提示用户重新下载等。
完整的示例代码如下:
```csharp
using System.IO.Compression;
public bool CheckZipFile(string filePath)
{
try
{
using (ZipArchive archive = ZipFile.OpenRead(filePath))
{
foreach (ZipArchiveEntry entry in archive.Entries)
{
if (!entry.FullName.EndsWith("/"))
{
entry.Open().Close();
}
}
}
return true;
}
catch (Exception ex)
{
// 记录日志等处理
return false;
}
}
```
C# 解压文件并且有解压进度和解压文件名
你可以使用 .NET Framework 中的 System.IO.Compression 命名空间来解压文件,同时使用 System.ComponentModel.BackgroundWorker 类来实现解压进度的显示。以下是一个示例代码:
```csharp
using System;
using System.IO;
using System.IO.Compression;
using System.ComponentModel;
public class Unzipper
{
private BackgroundWorker worker;
public Unzipper(BackgroundWorker worker)
{
this.worker = worker;
}
public void Unzip(string zipFilePath, string extractPath)
{
using (ZipArchive archive = ZipFile.OpenRead(zipFilePath))
{
int totalEntries = archive.Entries.Count;
int currentEntry = 0;
foreach (ZipArchiveEntry entry in archive.Entries)
{
string entryPath = Path.Combine(extractPath, entry.FullName);
worker.ReportProgress((int)((float)currentEntry / totalEntries * 100), entryPath);
currentEntry++;
if (entry.Name == "")
{
Directory.CreateDirectory(entryPath);
}
else
{
entry.ExtractToFile(entryPath, true);
}
}
}
}
}
```
在此示例中,Unzipper 类的 Unzip 方法接受两个参数:压缩文件的路径和解压路径。BackgroundWorker 参数用于报告解压进度。在 Unzip 方法中,我们首先使用 ZipFile.OpenRead 方法打开 zip 文件。然后,我们遍历压缩文件中的每个条目,并为每个条目报告解压进度。最后,我们使用 ZipArchiveEntry.ExtractToFile 方法将条目解压缩到指定的目录。
你可以在 BackgroundWorker 的 ProgressChanged 事件处理程序中来更新 UI,以显示解压进度和当前正在解压缩的文件名。
相关推荐











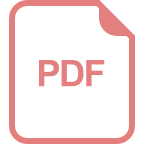
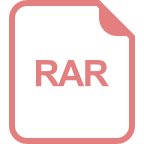