c语言实现bmp图片转NV12
时间: 2024-03-05 10:46:31 浏览: 116
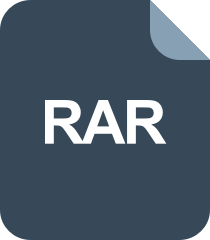
图片格式 NV21 转 RGB24 和 BMP图片源码(C语言)。
C语言可以通过读取BMP图片的像素数据,并将其转换为NV12格式。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
unsigned char blue;
unsigned char green;
unsigned char red;
} RGBPixel;
void bmpToNV12(const char* bmpFile, const char* nv12File) {
FILE* bmp = fopen(bmpFile, "rb");
if (!bmp) {
printf("Failed to open BMP file.\n");
return;
}
// 读取BMP文件头
fseek(bmp, 0, SEEK_SET);
unsigned char header[54];
fread(header, sizeof(unsigned char), 54, bmp);
// 获取图像宽度、高度和像素数据偏移量
int width = *(int*)&header[18];
int height = *(int*)&header[22];
int dataOffset = *(int*)&header[10];
// 计算每行像素数据所占字节数(包括填充字节)
int rowSize = ((width * 3 + 3) / 4) * 4;
// 分配内存保存像素数据
RGBPixel* pixels = (RGBPixel*)malloc(rowSize * height);
if (!pixels) {
printf("Failed to allocate memory.\n");
fclose(bmp);
return;
}
// 读取像素数据
fseek(bmp, dataOffset, SEEK_SET);
fread(pixels, sizeof(RGBPixel), width * height, bmp);
// 创建NV12文件
FILE* nv12 = fopen(nv12File, "wb");
if (!nv12) {
printf("Failed to create NV12 file.\n");
free(pixels);
fclose(bmp);
return;
}
// 写入NV12文件头
fwrite(header, sizeof(unsigned char), 54, nv12);
// 将RGB像素数据转换为YUV420(NV12)格式
unsigned char* yuvData = (unsigned char*)malloc(rowSize * height * 3 / 2);
if (!yuvData) {
printf("Failed to allocate memory.\n");
free(pixels);
fclose(bmp);
fclose(nv12);
return;
}
int yIndex = 0;
int uvIndex = width * height;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
RGBPixel pixel = pixels[i * width + j];
// 计算Y分量值
yuvData[yIndex++] = (unsigned char)(0.299 * pixel.red + 0.587 * pixel.green + 0.114 * pixel.blue);
// 每隔2个像素计算一次UV分量值
if (i % 2 == 0 && j % 2 == 0) {
// 计算U分量值
yuvData[uvIndex++] = (unsigned char)(-0.169 * pixel.red - 0.331 * pixel.green + 0.5 * pixel.blue + 128);
// 计算V分量值
yuvData[uvIndex++] = (unsigned char)(0.5 * pixel.red - 0.419 * pixel.green - 0.081 * pixel.blue + 128);
}
}
}
// 写入NV12像素数据
fwrite(yuvData, sizeof(unsigned char), rowSize * height * 3 / 2, nv12);
// 释放内存并关闭文件
free(pixels);
free(yuvData);
fclose(bmp);
fclose(nv12);
printf("BMP to NV12 conversion completed.\n");
}
int main() {
const char* bmpFile = "input.bmp";
const char* nv12File = "output.nv12";
bmpToNV12(bmpFile, nv12File);
return 0;
}
```
上述代码中,`bmpToNV12`函数用于将BMP图片转换为NV12格式。它首先读取BMP文件头,获取图像的宽度、高度和像素数据偏移量。然后根据每行像素数据所占字节数计算出行大小,并分配内存保存像素数据。接下来,它读取像素数据,并创建NV12文件。最后,它将RGB像素数据转换为YUV420(NV12)格式,并写入NV12文件。
你可以将需要转换的BMP图片路径传递给`bmpToNV12`函数的`bmpFile`参数,将转换后的NV12文件路径传递给`nv12File`参数。运行程序后,它将会将BMP图片转换为NV12格式并保存到指定路径的文件中。
阅读全文
相关推荐
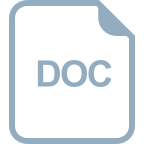
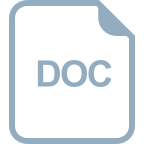
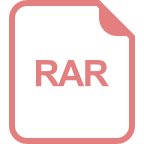
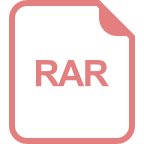
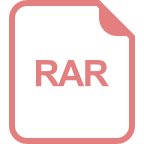
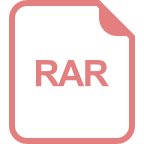
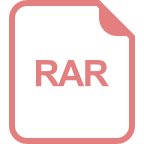
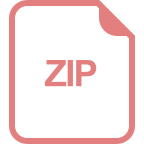
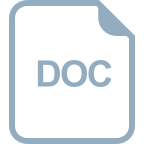
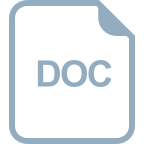
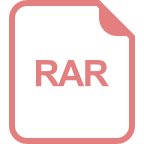
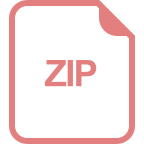
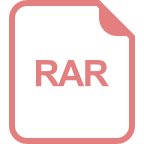
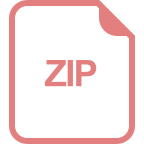
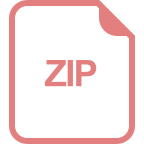