c#中从m个数中随机取出n个不重复的数
时间: 2024-09-18 19:10:12 浏览: 75
在 C# 中,为了从给定的一组 m 个数中随机选取 n 个不重复的数,你可以使用 `System.Linq` 的 `OrderByNew()` 或者 `Zip()` 函数结合 `Random` 类,这里提供一种基于排序的方法:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
public class Program
{
public static List<int> SelectUnique(int[] numbers, int n)
{
if (numbers.Length < n)
throw new ArgumentException("Not enough numbers to select without repetition.");
// 使用 Fisher-Yates 洗牌算法生成随机排列
Random random = new Random();
for (int i = numbers.Length - 1; i >= n; i--)
{
int j = random.Next(i + 1);
Swap(ref numbers[i], ref numbers[j]);
}
// 取前 n 个元素作为结果
return numbers.Take(n).ToList();
}
private static void Swap(ref int a, ref int b)
{
int temp = a;
a = b;
b = temp;
}
public static void Main(string[] args)
{
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9 }; // 例如这里有 m 个数字
int n = 3; // 需要取 n 个不重复的数
var selectedNumbers = SelectUnique(numbers, n);
Console.WriteLine("Selected unique numbers: " + string.Join(", ", selectedNumbers));
}
}
```
这个程序首先检查是否有可能选到不重复的 n 个数,然后对数组进行随机排序,最后取前 n 个元素。运行 `Main` 函数会显示随机选取的不重复的 n 个数。
阅读全文
相关推荐



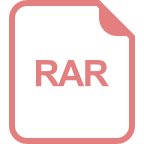












